Creating A Golang Map
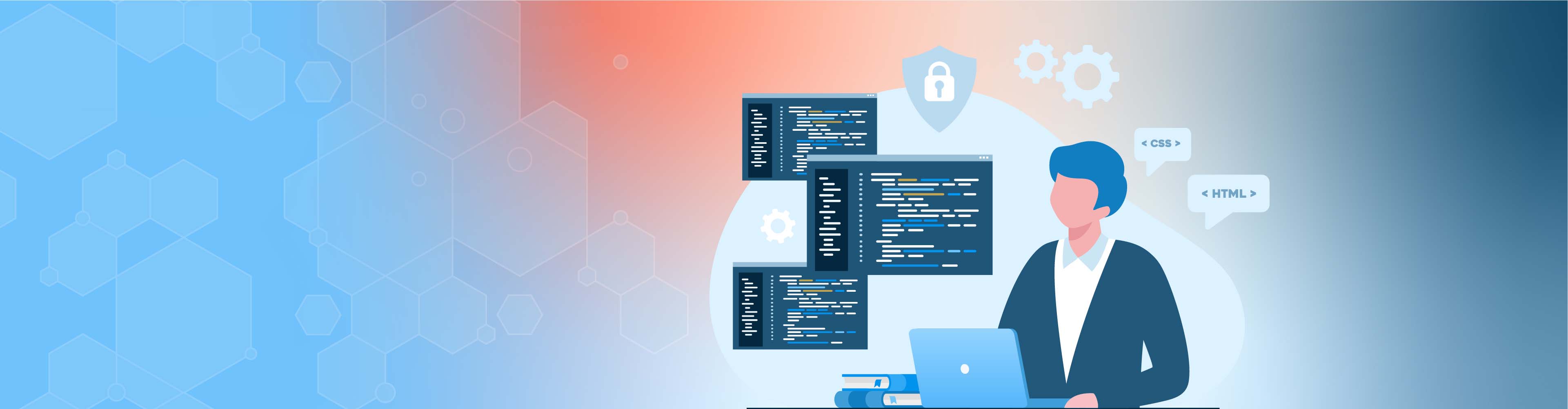
Creating A Golang Map
A map is a data structure that gives you an unordered collection of key & value pairs (In PHP, they are sometimes called an associative array, in java (hash table), or a dictionary in Python). The map is used to find the value based on the assigned key. They store the value based on the key in the map.
The advantage of the map is that it can quickly retrieve data according to the key. The key works similarly to an index and shows the value you assign to the key.
Mapping is implemented with a hash table, which provides faster views of the data item, and a value can be easily found through the key. Maps are cluttered collections, and the prediction of the order in which the key/value pairs can be retrieved is impossible. Each iteration on a map can return a varied order.
How does a Map work in the Go language?
As mentioned above, that the map is another data type that enables us to store unordered data types, such as hashes, with key-value pairs, then let’s talk about how the map works. It takes up little space, or we can just say that it is optimized. It's like the hash, like key-value pairs.
The value of the key on the map is always distinct in nature. Values on the map can either be unique or not. The main data type and the value data type must be the same. This means that the data types of all the keys on the map must be the same, and also the same thing for the data types of the values on the map. Here, the map will enable us to find various types of keys and values. This means that key and value data types may differ. We can use the key to access the properties of the map, which is very handy as it makes updating, deleting, and retrieving key .like map_name[key], here key can be key=10,100 very easy
How to create Map?
Simple method
Using method, you do not need the make() function for creating and initializing maps
Creating Map: Without so much stress you can create a map with the syntax below.
// An Empty map
map[Key_Type]Value_Type{}
// Map with key-value pair
map[Key_Type]Value_Type{key1: value1, ..., keyN: valueN}
For instance:
var mymap map[int]string
In the map, the empty value of the map is nil, and there are no keys in a nil map. If you try adding a key/value pair to a nil map, the compiler will encounter a runtime error.
Initializing map with map literals: The literal mapping is the easiest way to initialize the mapping with data, but the key-value pairs are separated by colons. If the last colon is not used, the compiler will report an error.
Example:
// Go program to illustrate how to
// create and initialize maps
package main
import "fmt"
func main()
{
// Creating and initializing empty map
// Using var keyword
var map_1 map[int]int
// Checking if the map is nil or not
if map_1 == nil
{
fmt.Println("True")
}
else
{
fmt.Println("False")
}
// Creating and initializing a map
// Using shorthand declaration and
// using map literals
map_2 := map[int]string{
90: "Dog",
91: "Cat",
92: "Cow",
93: "Bird",
94: "Rabbit",
}
fmt.Println("Map-2: ", map_2)
}
Output:
True
Map-2: map[90:Dog 91:Cat 92:Cow 93:Bird 94:Rabbit]
Using make() function: Maps can also be created with the make() function. This function is integrative, and you only need the passing of the map type in this method, and an initialized map will be returned.
Syntax:
make(map[Key_Type]Value_Type, initial_Capacity)
make(map[Key_Type]Value_Type)
Example:
// Go program to illustrate how to
// create and initialize a map
// Using make() function
package main
import "fmt"
func main()
{
// Creating a map
// Using make() function
var My_map = make(map[float64]string)
fmt.Println(My_map)
// As we already know that make() function
// always returns a map which is initialized
// So, we can add values in it
My_map[1.3] = "Rohit"
My_map[1.5] = "Sumit"
fmt.Println(My_map)
}
Output:
map[]
map[1.3:Rohit 1.5:Sumit]
Other essential functions that are available in Golang maps
Adding key-value pairs in the map
When creating maps, you can add key-value pairs in an initialized map with the syntax below
map_name[key]=value
In maps, if you try adding a key that has already been in existence, it will change the value of that key by simply updating or overriding the value.
How to retrieve a key-related value in the maps?
In the Golang maps, retrieving a value can be done by using the syntax given below.
map_name[key]
When the key is not present in the map, it will give an empty value to the map, i.e., nil. And if the key is present in the map, the value will be returned.
How to check whether a key exists on the map?
In maps, there is a way to check whether a key exists or not through the syntax below
// With value
// It will give the value and check the result
value, check_variable_name:= map_name[key]
or
// Without value using the blank identifier
// It will only give check result
_, check_variable_name:= map_name[key]
If the value of check_variable_name means that the key on the provided map is false and the value of check_variable_name is false, it means that the key on the provided map is not in existence.
How do I delete a key from the map?
On maps, you can delete the card key with the delete function (). It is a built-in function and does not return any value, and does nothing if the key is not present on the specified map. In this function, you only need to pass the map & key you want to delete in the map.
Syntax:
delete(map_name, key)
Leading companies trust Upstack to hire the tech experts they need, exactly when they need them! What are you waiting for? Get in touch today!