How To Debug Ruby Script
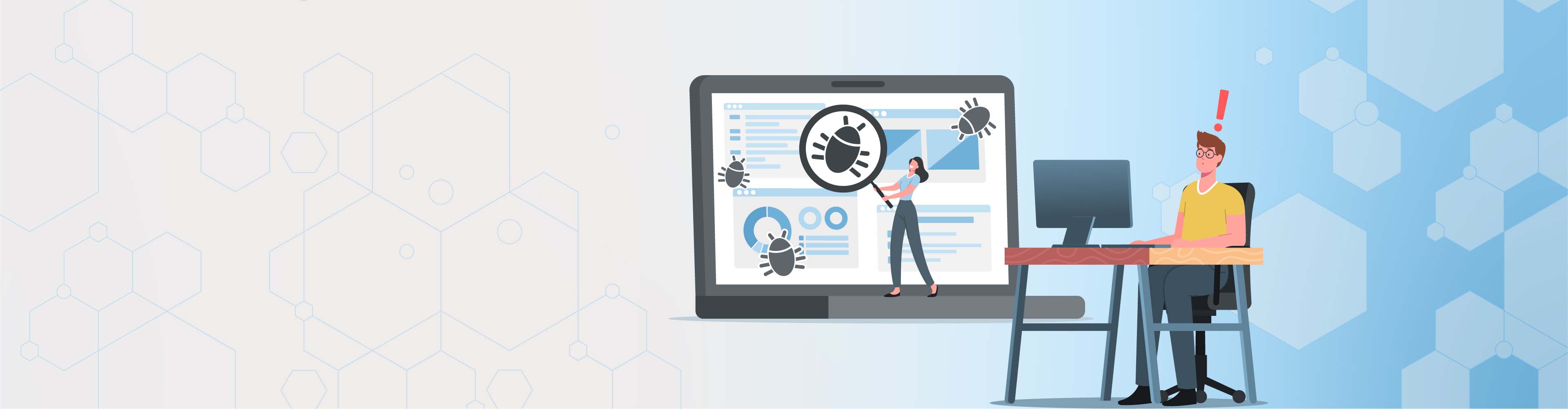
How To Debug Ruby Script
As a developer, we all have our own ways to find the source of problems. Some of us, probably many newbies, do this by strategically placing printed statements around the part we think is error-prone. In Ruby, you can load the entire contents of the data structures if necessary. This can be done by printing out the structure than the ".inspect" with puts. Or you can put "p" in front of the Ruby data structure for unloading its content. This will generate a data string.
Pry
You can install using the following command:
$ gem install pry
$ pry
Then add: require 'pry'; binding.pry into your code.
But in pry 0.12.2, some navigations commands such as next and break are not present. Although some gems offer this, an example is pry-byedebug
In Ruby:
ruby -rdebug myscript.rb
then,
b <line>: put break-point and n(ext) or s(tep) and c(ontinue)
p(uts) for display
(like perl debug)
In rails, you can launch the server with script/server – debugger and add a debugger in the code.
pry is an excellent repl choice then irb
It is essential you add require 'pry' to the source file and put a breakpoint in the same through the addition of binding.pry at the position, you want to check things
The moment your program hits the binding.pry line, you will be introduced into the pry repl with all your program context available to explore everything easily around, check all objects, modify the state and even tweak the code.
Puts debugging
While writing scripts, one of the easiest ways of debugging is “puts debugging” also known as printf debugging. It is as straightforward as calling the puts method with a variable whose value you want to know, e.g.
puts x
Representing an object using strings might not provide enough information, therefore you will usually want to utilize one of many variations. Calling the #inspect object usually provides enough information, such as
puts x.inspect
The p method offers a shortcut to save you some stress, this is also the same as the example given earlier:
p x
The pp library can be also be used to pretty-print output and handful while debugging more complicated objects:
require 'pp'
pp x
If you are unsure about your object type. Call #class:
p x.class
If you anticipate knowing interesting methods in this object. Try using:
p x.methods - Object.instance_methods
Vanilla Debugger: Byebug
Another useful gem is the byebug, it allows you to pause before executing your code to manually check to see what might not be right in the code. You won't need to keep running your code within your head, this gem gets you covered on all things.
You will get some debuggers equivalent to this irrespective of the language you choose. There are extremely handy.
If you want to use the byebug gem, install it, paste it into your code, and run it using the byebug method at the specific point where you think the error might occur:
# CLI
$ gem install byebug
# my_script2.rb
require 'byebug'
def buggy
byebug #<<<<<<<< where byebug will pause the first time
puts "I'm a buggy method!"
[1,2,5,0,1].each do |num|
1000/num
end
end
# IRB
> load 'my_script2.rb'
=> true
> buggy
[1, 9] in my_script2.rb
1: require 'byebug'
2:
3: def buggy
4: byebug
=> 5: puts "I'm a buggy method!"
6: [1,2,5,0,1].each do |num|
7: 1000/num
8: end
9: end
(byebug): # <<< this is the command prompt
As with IRB, switch to a Live Interactive Code (REPL) session when running a Byebug. You can then walk through your code examining the variables at each point along the way.
Useful commands:
the step takes you one step further in the program.
When ENTER is pressed it is often a continuation of the last command (for example, step).
p this will print the value of a variable when a variable is placed after it.
quit/q/exit leave Byebug.
The Ruby on Rails console (irb)
With an IRB console range, you can transfer your data to your own content. Accessing it is as straightforward as running rails console in an application from a selected terminal. You can create multiple types of queries to analyze and change exactly the type of data you want.
Passing the flag –sandbox while running rails console is also a fun hack. This allows "fake" changes to your data, but ultimately restores everything that has been changed. It may be useful to do this in practice to try to diagnose errors before determining to effect a change.
Logging
When you launch a local version of your rails app, you'll pretty clearly see a list of all things occurring in your terminal. However, this is very useful for identifying apps, parameters, and more when performing front end operations
The <%= debug(object) %> method
If you want to print out data on your front-end, this debugger is usually a lifesaver and you will find it useful every time.
As an assumption, if there is an @article available. You can add the codes written below:
<%= debug @article %>
<p>
<b>Title:</b>
<%= @article.title %>
</p>
The output will be something equivalent to the following:
--- !ruby/object Article
attributes:
updated_at: 2021-09-15 23:00:11
body: A useful guide that will walk you through ruby script debugging
title: How to debug ruby script
published: t
id: "1"
created_at: 2021-09-15 22:09:31
attributes_cache: {}
Title: How to debug ruby script
At Upstack we help you grow your business faster. We connect you with the top 1% developers in the world. Contact us NOW!