How To Install Firebase In Angular
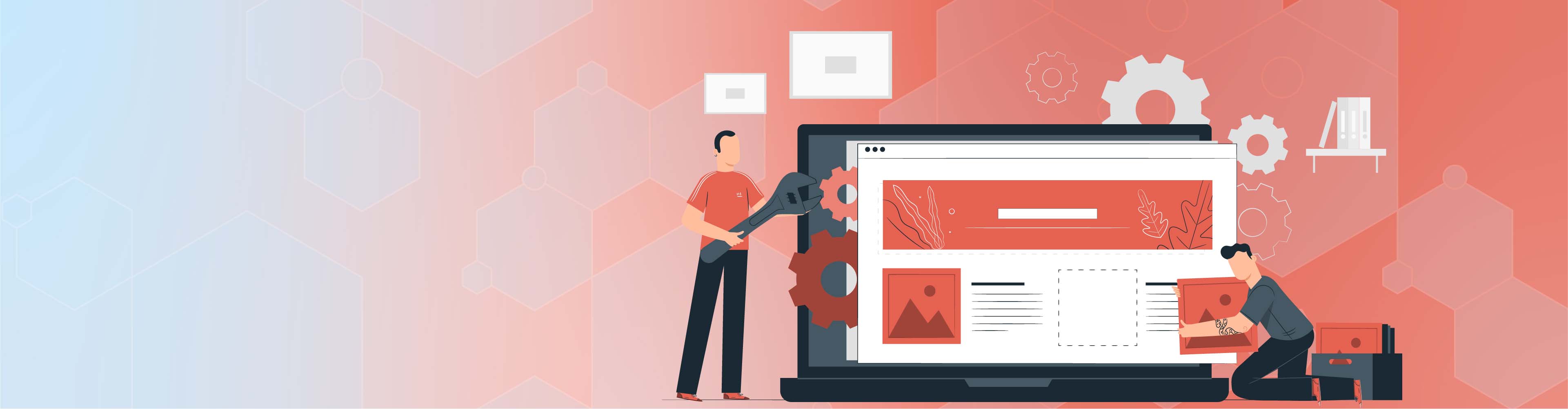
How To Install Firebase In Angular
Firebase is a platform (backend) specially designed to create web, Android, and IOS applications. It offers a real-time database, multiple APIs & authentication, and hosting platforms. In this article, we will cover some basic information and how you can add it to your web app and Android app.
History Of Firebase
Firebase was built by Envolve. Envolve is a startup established in 2011 by James Tamplin and Andrew Lee. The company offers API for developers to help them add an online chat feature to their websites. After the launch of its chat service, they discovered that evolve were used in the transmission of application data asides from chat messages. Developers utilized Envolve for synchronizing applications to distinguish the real-time architecture and the chat system. Although, the company has been separately established in 2011, however, was launched in 2012.
The first Firebase product was the Firebase real-time database. It is an API that synchronizes application data between Android, iOS, and web devices. It is stored in the Firebase cloud. Firebase’s real-time database then helps developers create real-time applications.
Why You Should Use A Firebase?
High User Engagement
An aspect of app development that is crucial is the ability to develop and interact with users over time. Firebase has many integrated functions to make your app more effective. With commercial applications, this is really the basis of how good Firebase is. Since the platform is highly useful for commercial apps, it is one of those reasons Firebase has been wonderful.
Simplified Application Development
Using Firebase, you can spend more time and energy developing an application that’s suited for your business. It features a solid operation and internal functions that are responsible for the Firebase Interface. Asides from developing applications easily, you will be focused on creating high-quality apps that are tailored to the interest of your users.
Integrated Analytics
One of the features you would love in Firebase is the Analytics dashboard. This feature is free and capable of reporting 500 types of events each having 25 attributes. The dashboard is very handy to observe and measure various behavior and attributes of users. Using this functionality, you will have a comprehensive understanding of people using your apps and consequently, it will help you to easily optimize your website later.
- Audience targeting: This allows the Firebase Console to identify custom audience segments according to device data, custom events, or user attributes. Then you can use the specified audiences with other Firebase properties to target new features.
- Integration with other services: There are a couple of services that can be useful for our business and we can integrate our app with them. Some of these services include Big Query, Firebase Notifications, Firebase Remote Configuration, Firebase CrashReporting, and Google Tag Manager.
Add Firebase To Your Android App
- Begin by creating a project in the Firebase console and select “Create New Project.”
- Select “Add Firebase to Android App” and follow the setup instructions.
- Once prompted, enter the name of your app package.
- It is essential that you input your app’s package name, you can only configure this when adding an application to your firebase project.
The debug signing certificate (SHA1) is needed for Dynamic Links, invites, and Google sign-In support in Authentication. If you want to add the certificate, head to the Android studio for your project, on the right side of the window select the Gradle tab, click Refresh, then head to project>Tasks>android>signingReport. In the Run tab, there will be a generation of MD5 and SHA1. Copy SHA1 and paste it into the Firebase console.
Finally, you need to download the google-services.json file. This file is downloadable at any time.
Next, you can add an SDK to incorporate the Firebase libraries into the project.
Add The SDK
If you want to add Firebase libraries to your project, it is necessary you complete several tasks to get your Android Studio Project ready. It is possible you have done this when adding Firebase to your application.
Integrate some rules to your build.gradle file to add the google-services plugin:
buildscript {
// ...
dependencies {
// ...
classpath 'com.google.gms:google-services:3.0.0'
}
}
Then, in the Gradle file of your module (app/build.gradle), put the line of the plugin at the end of the file to activate the Gradle plugin:
apply plugin: 'com.android.application'
android {
// ...
}
dependencies {
// ...
compile 'com.google.firebase:firebase-core:9.4.0'
}
// Add to the end
Add plugin: 'com.google.gms.google-services'
Add Firebase To Your Web App
In your Firebase project, select the gear symbol closer to "Project Overview" and you will your project settings. In the "Your Apps" section on the "General" tab, select the </> button. This will bring up a pop-up window named “Add Firebase to your web application.” Just copy the config object from this page.
Config object
export const environment = {
production: false,
firebaseConfig : {
apiKey: 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx',
authDomain: 'file-upload-demo-for-articles.firebaseapp.com',
databaseURL: 'https://file-upload-demo-for-articles.firebaseio.com',
projectId: 'file-upload-demo-for-articles',
storageBucket: 'file-upload-demo-for-articles.appspot.com',
messagingSenderId: '4031356181',
appId: '1:4031356181:web:80d30bf4f9aedd9c6b9d1c',
measurementId: 'G-J55PBZ43G5'
}
};
Install @angular/fire Via NPM
Install @angular/fire and firebase as dependencies in your Angular Project.
npm install firebase @angular/fire
Add @angular/fire To The App Module
Here, the Firebase modules can be imported into Angular. The only module you will be needing now is the AngularFireModule. The other modules can be added or removed according to your needs.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
// 1. Import the libs you need
import { AngularFireModule } from '@angular/fire';
import { AngularFirestoreModule } from '@angular/fire/firestore';
import { AngularFireStorageModule } from '@angular/fire/storage';
import { AngularFireAuthModule } from '@angular/fire/auth';
// 2. Add your credentials from step 1
const config = {
apiKey: '<your-key>',
authDomain: '<your-project-authdomain>',
databaseURL: '<your-database-URL>',
projectId: '<your-project-id>',
storageBucket: '<your-storage-bucket>',
messagingSenderId: '<your-messaging-sender-id>'
};
@NgModule({
imports: [
BrowserModule,
// 3. Initialize
AngularFireModule.initializeApp(config),
AngularFirestoreModule, // firestore
AngularFireAuthModule, // auth
AngularFireStorageModule // storage
],
declarations: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule {}
Upstack gives you the ability to search the perfect software dev for your project by skill, location, experience level and more. Contact us!