How To Print An Array In Java
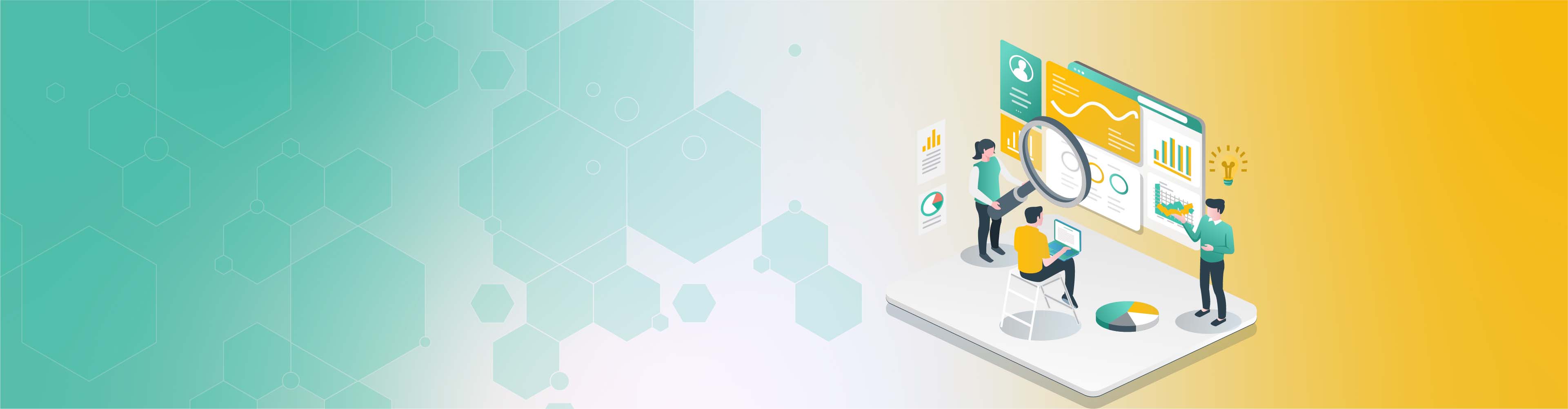
How To Print An Array In Java
Essentially, an array is a data structure in which we can store elements of a similar type. For instance, some array saves different integers; there are multiple lines in a string array, and so on. Hence, if you have a dataset with a lot of data, you may need to print it in order to view it using a print array in Java. There are different ways to print an array in Java. You can use any of these methods to print the array. The methods that are listed below have different examples to make you have a comprehensive understanding of the methods.
Printing an array in Java is not as easy as in other programming languages. The default method for printing objects will not produce the expected results. To avoid this, there are various methods we can use to print the values in the array. We will look at some examples of code to show how this can be done.
Print an Array in Java using Arrays.toString()
The array class in java.util package is pre-defined. It contains many predefined array-related methods and provides the solution for a lot of array tasks. Arrays class provides ToString() mode to display the elements of the specified array in string format.
So, when we need to display a one-dimensional set of primitive data types, it is advisable you use the Arrays.toString() method. The Arrays.toString() method arranges the elements in square brackets [] when displayed.
Example of a Java program that uses the Arrays.toString() method to print an array:
// import Arrays class
import java.util.Arrays;
public class DisplayArray {
public static void main(String[] args) {
// declare and initialize an array
int arr[] = {10, 20, 30, 40, 50};
// display the array using Arrays.toString()
System.out.print(Arrays.toString(arr));
}
}
Output:
[10, 20, 30, 40, 50]
Using For Loop
This is by far the easiest way to print or iterate through an array in any programming language; when asked to print an array as a programmer, the first thing you must do is start writing the loop. You can use for loop to iterate over the elements of the array.
Below is a program that demonstrates the use of "for" loops in Java.
public class Main
{
public static void main(String[] args) {
Integer[] myArray = {10,20,30,40,50};
System.out.println("The elements in the array are:");
for(int i =0; i<5;i++) //iterate through every array element
System.out.print(myArray[i] + " "); //print the array element
}
}
Output:
The element in the arrays are:
10 20 30 40 50 60
The "for" loop traverses every element in Java, so you need to know when to stop. So, to use a for loop to access the elements of an array, you need to give it a counter that tells you the number of times it needs to loop.
Using For-Each Loop
You can also access the array elements by using Java for-Each loop. The application is similar to For-loop through each element of the matrix, but the syntax for For-Each is slightly different.
Below is an example of program implementation:
public class Main
{
public static void main(String[] args) {
Integer myArray[]={10,20,30,40,50};
System.out.println("The elements in the array are:");
for(Integer i:myArray) //for each loop to print array elements
System.out.print(i + " ");
}
}
Output:
The elements in the array are:
10 20 30 40 50 60
When using for-Each, different from for loops, there is no need for a counter. This loop traverses all elements of the array until the end of the array is reached, and all elements can be accessed. The 'forEach' loop is dedicated to accessing the elements of the array.
DeepToString
The "DeepToString" used in printing a two-dimensional array is the same as the "toString" method mentioned above. If you only use "toString" because the structure is an array within an array of multidimensional arrays, only the element addresses are printed.
Therefore, we use the function "deepToString" of class Arrays to print multi-dimensional array elements.
The following program shows the "deepToString" method.
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
//2D array of 3x3 dimensions
int[][] array_2d = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
System.out.println("Two-dimensional Array is as follows:");
System.out.println(Arrays.deepToString(array_2d)); //convert 2d array to string and display
}
Two-dimensional array is as follows:
[[1,2,3], [4,5,6], [7,8,9]]
Java Iterator interface
Java Iterator is an interface that is part of java.util package. Iterator objects can be created by calling the iterator () method. It exists in the collection interface and an iterator is returned.
Example iterator interface
In the code example below, we declare an array while initializing the elements in it. Firstly, we use the Arrays.asList () method to convert the given array to a list, as the iterator enables us to iterate through the collection, and then we call the iterator () method of the collection class.
import java.util.Arrays;
import java.util.Iterator;
public class PrintArrayExample6
{
public static void main(String[] args)
{
//declaration and initialization of an array of Double type
Double[] array = { 1.5, 2.6, 3.7, 4.8, 5.9};
//returns an iterator
Iterator<Double> it = Arrays.asList(array).iterator();
while(itr.hasNext()) //returns a boolean value
{
System.out.println(itr.next());
}
}
}
Output:
1.5
2.6
3.7
4.8
5.9
Java Stream API
Java Stream is an on-demand computed data structure. It doesn’t have any data storage. Its works are based on source data like array and collection. The Java Stream API is used for internal iteration implementations. It offers several functions, for example: Sequential and parallel execution.
Java stream() method
Java stream () is a Java arrays class method, which is part of java.util package. It is used to obtain the matrix sequence stream.
Syntax:
public static <T>Stream stream(T[] array)
Where T is the array type. An array would be accepted if its elements will be converted to sequence flow. It returns a continuous IntStream with the specified array as the source.
Are you interested in starting a new project with the top 1% talent worldwide? Work with Upstack!