How To Use FlatList React Native
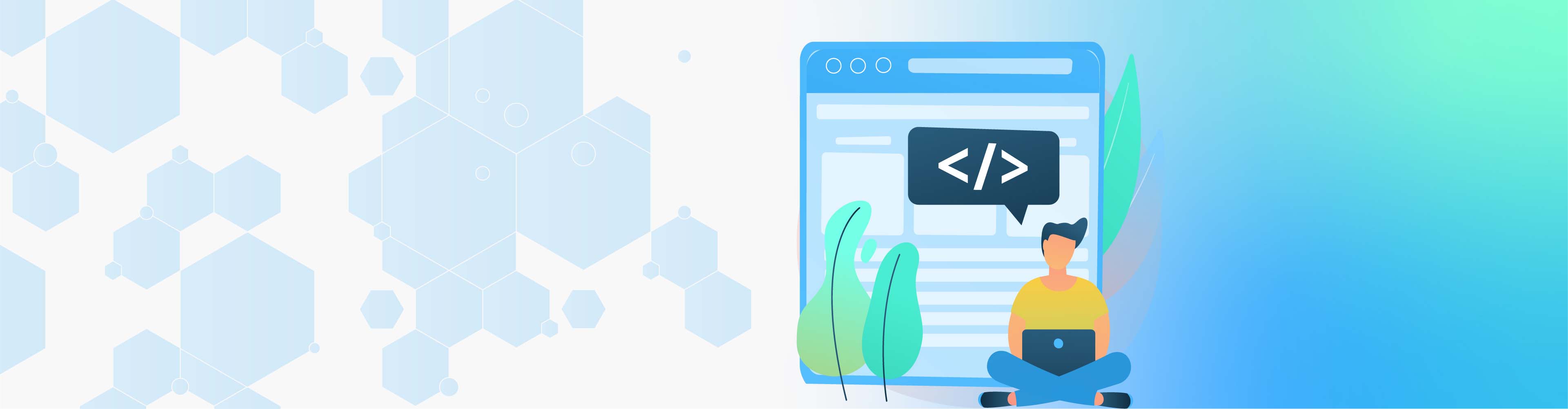
How To Use FlatList React Native
FlatList is created to handle the display of a list that has the same data objects. The display of each element has the same format and utilizes a common style sheet. The data is structured and scrollable. FlatList utilizes the ScrollView component for rendering elements but works immediately without pure ScrollView performance issues and has a few additional features that can create a better user interface and simplify the development process.
While displaying a long list of scrollable items, that’s when the FlatList component becomes useful.
The React Native FlatList component requires two support items: data and renderItem.
The data prop is the data that will be shown in the FlatList component. The data type can be any type, from an array that starts with a number to an array filled with JSON objects that you get through the API.
The renderItem prop as a function takes the item object of each data prop and lists it in the component. Suppose you want to display your data in a special style. In that case, you can do so in renderItem or refer to the method the style creates for you by using renderItem prop (for example renderItem = {this.RenderStyling} ).
Props in FlatList:
- renderItem: This prop is useful for rendering data into the list
- data: This is an array of data
- ItemSeparatorComponent: Used for rendering between items
- ListEmptyComponent: The list is rendered, provided it is empty.
- ListFooterComponent: It is rendered at the footer side of the item.
- ListFooterComponentStyle: used to set the style of the ListFooterComponent internal view.
- ListHeaderComponent: Renders at the footer area of all items.
- ListHeaderComponentStyle: used to format the built-in ListHeaderComponent view.
- columnWrapperStyle: This is an optional wrapping style for lines with multiple items.
- extraData: is the attribute that prompts the list re-rendering.
- getItemLayout: This is an optional optimization; it allows you to bypass dynamic content measurement if you know the item size.
- Horizontal: If true, the element is rendered horizontally rather than vertically.
- initialNumToRender: indicates the number of elements that should be rendered in the first batch.
- initialScrollIndex: If specified, the system will not start from the top element but the initialScrollIndex element.
- Inverted: Reverses the scroll direction.
- keyExtractor: the unique key used to extract the specified item.
- numColumns: for rendering different columns.
- onEndReached: called as soon as the scrolling position enters the rendered content.
- onEndReachedThreshold: let us know the distance from the end.
- onRefresh: If specified, a standard RefreshControl is added.
- onViewableItemsChanged: is given when row visibility changes.
- progressViewOffset: sets if load offset is required. Only present on Android.
- refreshing: set to true during the wait for newly refreshed data.
- removeClippedSubviews: This can improve the performance of large lists. The default value on Android is true.
- viewabilityConfigCallbackPairs: Displays a list of pairs.
How to use the FlatList component
Using the FlatList component requires that you use the two things below:
- The list of data to be rendered.
- Component for rendering each data item in the list. (We can utilize integrated components or create customized components.)
How to create a data list
As mentioned earlier, there is a need to have a list of data
In this example, we will create a list with different users
const users = [
{ id: 1, name: "Mark Angel", age: 30, profession: "Accountant" }
{ id: 2, name: "Abby Jones", age: 35, profession: "Lawyer" },
{ id: 3, name: "Theresa Williams", age: 22, profession: "Doctor" }
];
How to create a custom component
Then we can now create a component usable for rendering user data from the list of data.
Following is the example of a custom user component that has been created:
function User({ data }) {
const { name, age, profession } = data;
return (
<div
style={{
boxShadow: "0 0 2px rgba(0,0,0, 0.3)",
margin: "5px",
padding: "10px 25px",
fontSize: "1.2rem"
}}
>
<p>Name: {name}</p>
<p>Age: {age}</p>
<p>Profession: {profession}</p>
</div>
);
}
The user component takes prop data that consist of the data of an individual user. In a user component, we parse various attributes of a data object and then render those attributes. In addition, some CSS styles have been included on the div element (wrapper) in the user component.
Using the FlatList component
The FlatList component is now ready for use. Therefore, there is a need to pass the three props below to the FlatList component.
- data - Contains the list of data that will be rendered.
- renderItem is a function that requires returning the component that will display the data in the list.
- keyExtractor – This is a prop responsible for taking an individual item of our data as an argument and returning a value used as a key. The id will be used as a key
Next, we can create a FlatListDemo component that can be reused and also use the FlatList component:
const FlatListDemo = ({ dataList, RenderComponent }) => {
return (
<View>
<FlatList
data={dataList}
renderItem={(obj) => <RenderComponent data={obj.item} />}
keyExtractor={(item) => item.id}
/>
</View>
);
};
FlatListDemo component receives the two props below:
- dataList – This is a list that contains the data to be rendered.
- RenderComponent – The function of this component is to render the data that are passed as a dataList.
The renderItem prop function will receive an object containing a key item. The value of this important item will be an object of a single user from the user list. This item property will be assigned to one RenderComponent.
Use the FlatListDemo component
FlatListDemo component can be used as follows:
<FlatListDemo
dataList={users}
RenderComponent={User}
/>
We are growth pros. CONTACT US so we can help you scale your team with top 1% talent worldwide!