Iterating On Objects In React
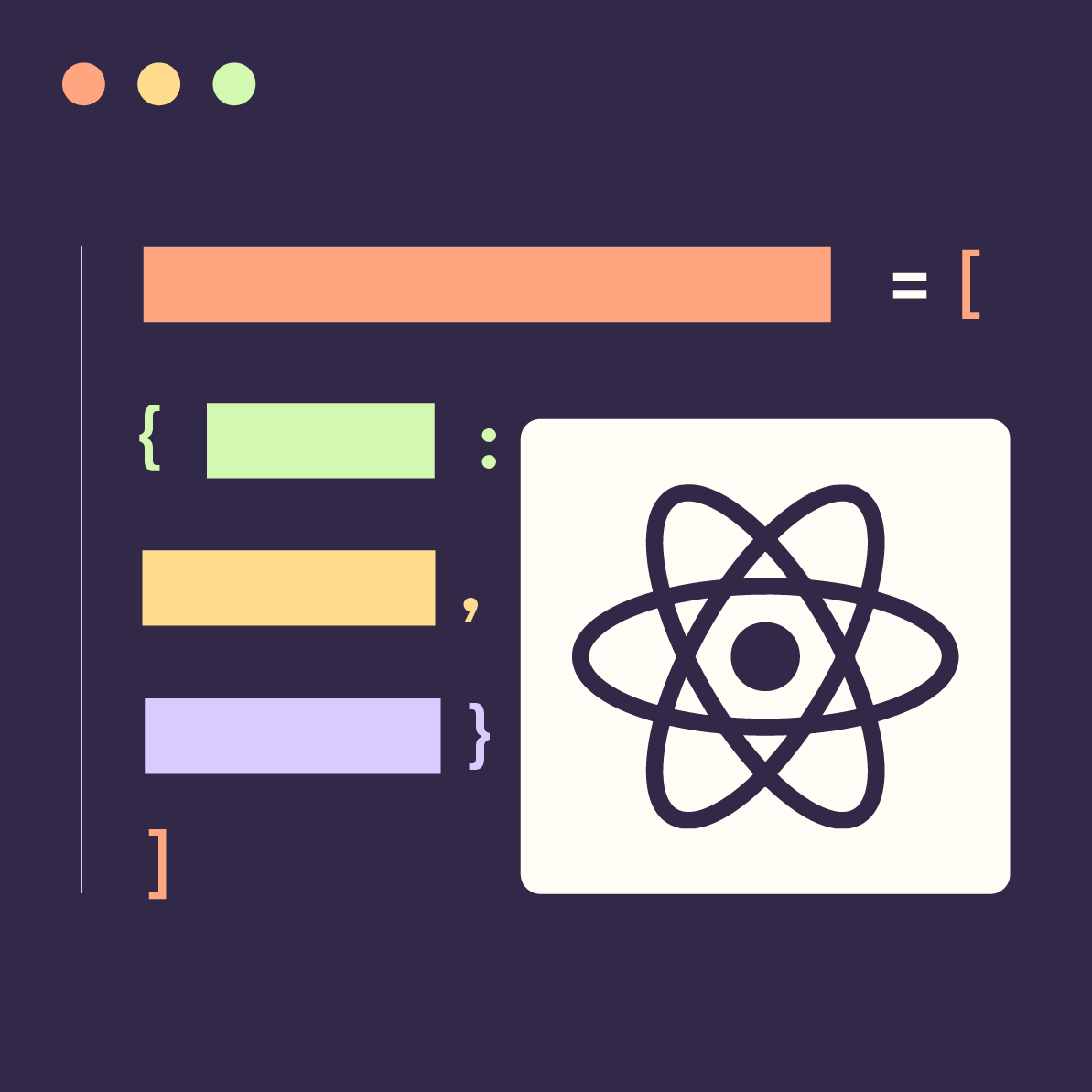
Iterating On Objects In React
Iterating on objects in React is the same as in JavaScript or any other framework or library that uses JavaScript. This article will first provide a brief overview of how one loops over the object data structure and then discuss which methods are most typically used in React development and why.
Iterating On Objects
While Arrays have the traditional for loop and the Array.forEach method, Objects have their own version: the for..in loop.
The for..in loop will iterate on an object’s properties. See the example below for how we might use this to loop over an object’s enumerable properties to access its values.
const foobar = {
foo: 3,
bar: 'seven',
fizz: [1, 'b', false]
}
for (val in foobar) {
console.log(`foobar[${val}] = ${foobar[val]}`);
}
But perhaps we wish to chain together some transformations on an object – its keys or its values.
In these cases, we can use the following Object built-in methods to get an array of what we need; Object.keys and Object.values, respectively.
console.log(Object.keys(foobar)); // ['foo', 'bar', 'fizz']
console.log(Object.values(foobar)); // [3, 'seven', [1, 'b', false]]
Applying this to React
Generally speaking, when we’re working with React we are taking data and using that to render something to the screen for the user. In this situation, it’s typical to transform what we need from the object into an array. Arrays can almost immediately be transformed into JSX with a simple map, provided the data we map over has an accessible key, so they’re a much friendlier data type when trying to display data for the user.
Now, we could use for..in to populate a new Array variable, or we could directly pipe what we need from the object into a map function. To this purpose, using Object.keys or Object.values is much more desirable. See for yourself:
// Using for..in
const MovieList = ( ) => {
const movies = {
batman: { title: 'The Batman', year: 2022 },
joker: { title: 'Joker', year: 2019 },
parasite: { title: 'Parasite', year: 2019 },
};
let movieItems = [ ];
for (movieKey in movies) {
movieItems.push({ id: movieKey, ...movies[movieKey]});
}
movieItems = movieItems.map(movie => (
<li key={movie.id}>{ movie.title }</li>
));
return (
<ul>{ movieItems }</ul>
);
}
// Using Object.keys
const MovieList = ( ) => {
const movies = {
batman: { title: 'The Batman', year: 2022 },
joker: { title: 'Joker', year: 2019 },
parasite: { title: 'Parasite', year: 2019 },
};
const movieItems = Object.keys(movies).map(movieKey => ({
id: movieKey,
...movies[movieKey]
})).map(movie => (
<li key={movie.id}>{ movie.title }</li>
));
return (
<ul>{ movieItems }</ul>
);
}
In the above MovieList component, we were able to reduce the boilerplate code without sacrificing readability when using Object.keys instead of for..in.
Note we could have used Object.values, as well, perhaps using the movie title as the key instead. I opted for the latter so that we could have a more reliable key. Object properties have uniqueness built-in, whereas it’s possible for two of the values to have the same title property value.