Node JS Express Tutorial
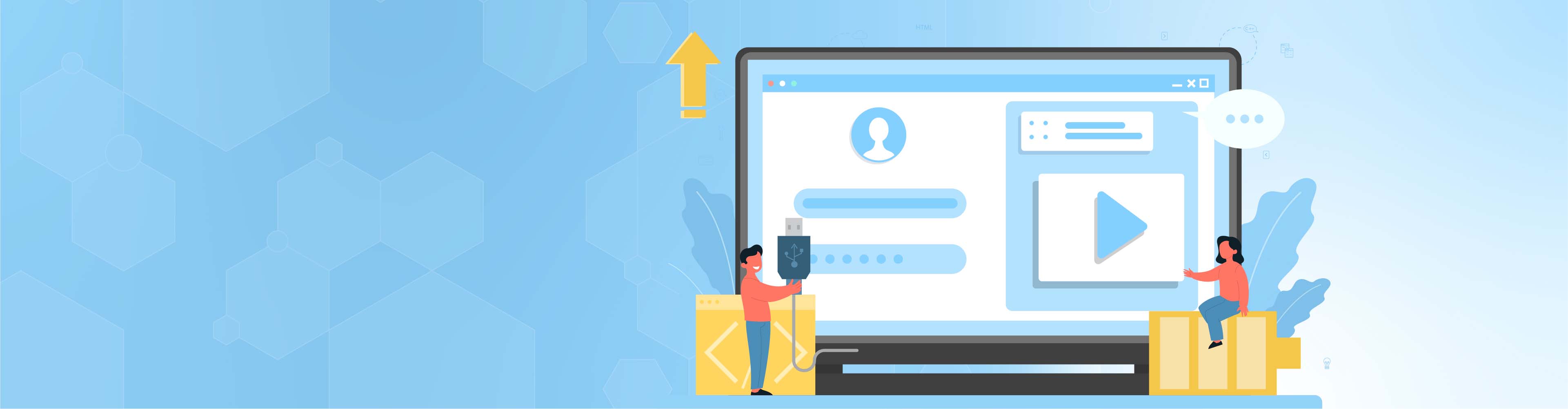
Node JS Express Tutorial
Express is a backend web framework that is built on top of Node.js. It is the standard framework for building and managing web servers via NodeJS.
Express JS adds extra flexibility to an application, in addition to a huge choice of modules available on the repository that you can plug directly into Express as required. This integration improves seamless data flow in linking servers and routes in server-side applications.
This framework was developed by TJ Holowaychuk and was made available in the market on the 22nd day of May 2010.
Express is currently managed by the Node.js foundation incubator. However, it used to be under the care of IBM via StrongLoop.
The main responsibility of Express is to control the backend part of the MEAN stack. Mean stack refers to the software stack that lets developers build dynamic websites and web applications in the market.
The full meaning of the MEAN is
- MongoDB – The standard NoSQL database
- Express.js – The default web applications framework
- Angular.js – The JavaScript MVC framework used for web applications
- Node.js – Framework used for scalable server-side and networking applications.
The framework of Express.js is very flexible and requires minimal stress to build an application capable of handling multiple requests.
How to install Express.js
Before you can install Express.js on your computer or system, you must first install Node.js. If you don't have Node.js installed, then you have to do so before attempting to install Express.
Now you have Node.js installed, the first step to installing express is to create a project directory. Afterward, you can create a package.json file that will hold the project dependencies for the time being.
Below is the code to perform the same;
npm init
It is ready for system installation now. To install it globally, use the below command:
npm install -g express
If your choice is to install locally in a folder, you should use this command:
npm install express –save
Now the installation process is done and dusted, let's dive right into explaining some Express.js concepts.
Hello world Example
Following is a very basic Express app that starts a server and listens on port 8081 for a connection. This app responds with Hello World! for requests to the homepage.
For every other path, it will respond with a 404 Not Found.
var express = require('express');
var app = express();
app.get('/', function (req, res) {
res.send('Hello World');
})
var server = app.listen(8081, function () {
var host = server.address().address
var port = server.address().port
console.log("Example app listening at http://%s:%s", host, port)
})
Save the above code in a file named server.js and run it with the following command.
$ node server.js
You will see the following output −
Example app listening at http://0.0.0.0:8081
Fundamental Concepts in Express.js
Routing and HTTP Methods
Routing is the term used to describe the process of dictating the specific behavior of a particular application.
It is all about the application responds to a client to a particular route, path, or URI along with a specific HTTP request.
Each route can be made up of multiple handler functions, executed when the client makes inquiries for a specific route.
Here is the routing structure in Express.
app.METHOD(PATH, HANDLER)
Code clarification:
the app is just an instance of Express.js. You can use any variable of your choice.
- METHOD is an HTTP request method such as to get, set, put, delete, etc.
- PATH is the route to the server for a specific webpage
- HANDLER is the callback function that is executed when the matching route is found.
There are four major HTTP methods, these are GET, POST, PUT and DELETE.
var express = require('express');
const app = express();
app.use(express.json());
app.get('/', function (req, res) {
console.log("GET Request Received");
res.send('
<h2 style= "font-family: Malgun Gothic; color: blue; "> Welcome to Edureka's Express.js Tutorial!/h2>');
})
app.post('/addcourse', function (req, res) {
console.log("POST Request Received");
res.send('
<h2 style="font-family: Malgun Gothic; color: green; ">A course new Course is Added!</h2>
');
})
app.delete('/delete', function (req, res) {
console.log("DELETE Request Received");
res.send('
<h2 style="font-family: Malgun Gothic; color: darkred;">A Course has been Deleted!!</h2>
');
})
app.get('/course', function (req, res) {
console.log("GET Request received for /course URI");
res.send('
<h2 style="font-family: Malgun Gothic; color:blue;">This is an Available Course!</h2>
');
})
//PORT ENVIRONMENT VARIABLE
const port = process.env.PORT || 8080;
app.listen(port, () => console.log(`Listening on port ${port}..`));
REST APIs
The full meaning of REST is Representational State Transfer. It’s an architectural style with an approach for communications motive. This is vastly utilized in numerous web services development.
An application program interface (API) works with the HTTP requests to GET, PUT POST, and DELETE the data over WWW.
Express is the perfect choice for a server in this context because it was built on the middle module of Node.js, by the name connect.
Express erases the methods or processes involved in making and revealing APIs for the server to communicate with the server application, posing as a client.
The connect module uses the HTTP module, which implies that working with connect-based middleware modules grants you easy integration with Express.js.
Cookies
Small and extremely important data that are stored in users’ computer.
These information files hold key details about the amount of data specific to a particular user and the type of websites he visits.
Anytime a user uses the internet and loads a page, data stored locally are sent back to the website, which processes the data and uses it to recognize the user. Basic elements of cookie include the following:
- Name
- Value
- Zero or more attributes in key-value format. These attributes can include cookie’s expiration, domain, and flags, etc.
Before you can use cookies in Express, you will need to have the cookie-parser middleware installed through mom into the node_modules folder already in your project folder.
Below is the code to execute the command
mom install cookie-parser
The next step now is for you to import the cookie parser into your application. Below is the code to do execute the command:
var express = require('express');
var cookieParser = require('cookie-parser');
var app = express();
app.use(cookieParser());
With the crash introduction to Express JS above, you should have some basic knowledge of how this web framework works and how you can set up your first Node JS Express Hello World project.
Drop us a line and let’s get in touch - embrace remote working through Upstack!