Object-Oriented Programming With C# - Abstract And Sealed Classes
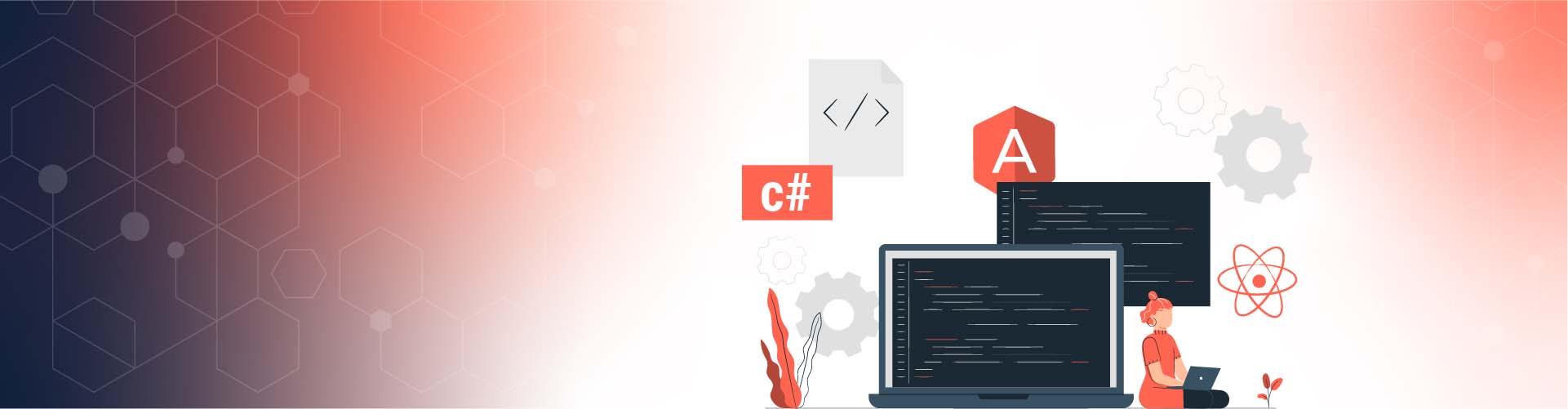
Object-Oriented Programming With C# - Abstract And Sealed Classes
- Topics of Discussion
- Abstract Classes and Methods
- Sealed Classes and Methods
1. Topics of Discussion
In this tutorial, we are going to talk about abstract and sealed classes. So, let's have some fun.
2. Abstract Classes and Methods
C#, along with other OOP languages, allows you to mark your class as abstract using the abstract keyword. When a class is marked as abstract, you cannot explicitly instantiate it. C# will generate a constructor for it though since abstract classes usually get inherited by other classes and as you remember, the parent class needs to get instantiated first. C# takes care of this for you, so you don't need to worry about it.
Note that you can still define your own explicit constructors in abstract classes. You just need to remember to invoke them in child classes using the base keyword.
Abstract classes are usually used alongside abstract methods. An abstract method is different from a regular method because of the following two things:
- it is marked as abstract by using the abstract keyword
- the method has no implementation and must be overridden in all the classes that inherit this abstract class
As you can remember from the previous tutorial, I mentioned that interface methods are public and abstract by default. Let's modify our Person class by making it abstract and also mark the SayHello( ) method as abstract:
public abstract class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string Nationality { get; set; }
public string SocialSecurityNumber { get; set; }
public Person(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber)
{
FirstName = firstName;
LastName = lastName;
DateOfBirth = dateOfBirth;
Nationality = nationality;
SocialSecurityNumber = socialSecurityNumber;
}
public abstract void SayHello();
}
And now, our Student class which inherits from Person needs to be modified as follows:
public class Student : Person
{
public Student(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber) : base(firstName, lastName, dateOfBirth, nationality, socialSecurityNumber)
{
}
public string College { get; set; }
public bool HasGraduated { get; set; }
public override void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName} and I am a student at {College}");
}
}
As you can see, we need to use the override keyword on abstract methods which need implementation.
3. Sealed Classes and Methods
The sealed keyword in C# has one purpose: preventing a class from being inherited by another (when marking a class as sealed) or preventing a method from being overridden in a child class. Of course, abstract classes cannot be sealed.
The idea behind sealing classes and methods is to prevent someone from accidentally changing some behavior that should not really be changed. For example, say you have a method that initializes something and the initialization order is important. If someone were to modify that method in a child class by overriding it, then the application might not work as expected. When invoking the method. Sealing the method, or the class itself if you don't want someone to actually inherit from it, will prevent any faulty behavior in your app.
And that about covers it for this tutorial. In the next one, we are going to conclude the course. See you then.
References
At Upstack we help you grow your business faster. Upstack connects you with the top 1% C# developers in the world. Contact us NOW!