Object Oriented Programming with C# - Abstraction and Encapsulation
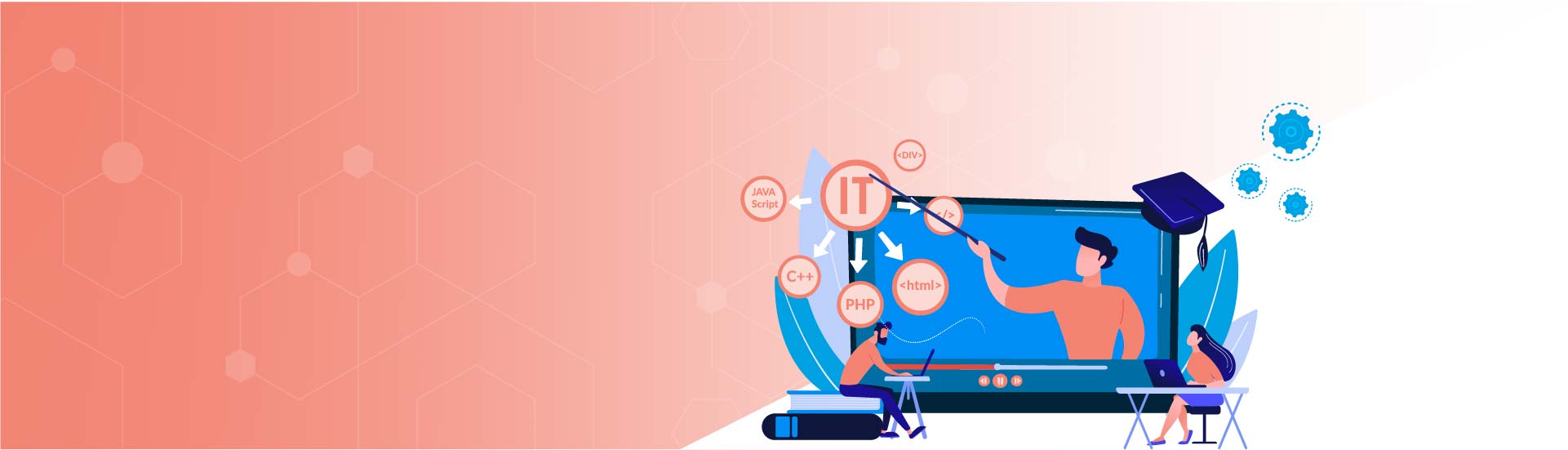
Object Oriented Programming with C# - Abstraction and Encapsulation
- Topics of discussion
- Abstraction and encapsulation in C#
- Encapsulating constructors
1. Topics of discussion
In this tutorial, we are going to discuss the abstraction and encapsulation OOP concepts and how they are implemented in C#. So, let's have some fun.
2. Abstraction and encapsulation in C#
Abstraction and encapsulation are the two OOP concepts that we have encountered so far in these tutorials. As you remember, abstraction is the art of making sure your classes are abstract representations of real-life objects and encapsulation is the process in which you do not allow your program direct access to class attributes. Instead, you use accessor methods.
Let's take another look at the Person class we have been using so far:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string Nationality { get; set; }
public string SocialSecurityNumber { get; set; }
public Person(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber)
{
FirstName = firstName;
LastName = lastName;
DateOfBirth = dateOfBirth;
Nationality = nationality;
SocialSecurityNumber = socialSecurityNumber;
}
public void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName}");
}
}
Abstraction was used to make sure we only the most important aspects that describe a person in this class. Of course, this is a very abstract representation of a person, but you get the idea. Encapsulation was used when creating the class attributes. Let's review the attribute signature:
public data_type VariableName { get; set; }
The get; and set; markers are used to tell C# to generate two methods:
- a getter method that allows you to gain access to the value of the variable
- a setter method that allows you to change the value of the variable
The public keyword is known as an access modifier denoting the access level used on the generated methods. C# offers a bunch of different access levels for your variables and methods:
- public - a variable or method declared as public is visible anywhere in the project
- private - a variable or method declared as private is only accessible in the class it is defined in; this is the default access level, meaning that if no access modifier is used on a variable or method, C# will interpret it as private
- protected - a variable or method declared as protected will be visible in the class it is defined in and in any class which inherit from said class
- internal - a variable or method declared as internal will be visible in any code located in the same assembly; it will not be visible outside that assembly though
- protected internal - same as internal, with the protected internal method or variable also being visible in classes that inherit that class, regardless of the assembly the child classes are located in
- private protected - similar to private, but the private protected class/method will also be visible in child classes (classes that inherit from the one that contains the private protected element)
You can also mark the get or set attributes as private:
public data_type VariableName { get; private set; }
The set method is still accessible in the class that defines it, but it will not be available outside of the class.
3. Encapsulating constructors
Access modifiers can also be used on constructors. The rules are the same as the ones mentioned above and the effect is similar. If you define a constructor as anything other than public, then there will be limits on where you can use that constructor. Here are some examples of constructors with access modifiers:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string Nationality { get; set; }
public string SocialSecurityNumber { get; set; }
private Person() { }
protected Person(string firstName)
{
}
public Person(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber)
{
FirstName = firstName;
LastName = lastName;
DateOfBirth = dateOfBirth;
Nationality = nationality;
SocialSecurityNumber = socialSecurityNumber;
}
public void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName}");
}
}
In the example above, the parameterless constructor would only be visible in the Person class while the constructor with only one parameter would be visible in the Person class and any and all classes that inherit the Person class.
And that about covers it for this tutorial. In the next one, we are going to discuss polymorphism with method overloading. See you then.
Great news! We match words with deeds - and the truth is that... We can connect you with the top 1% developers in the world! Let's do some magic together! Contact us NOW!