Object Oriented Programming with C# - Classes and Objects
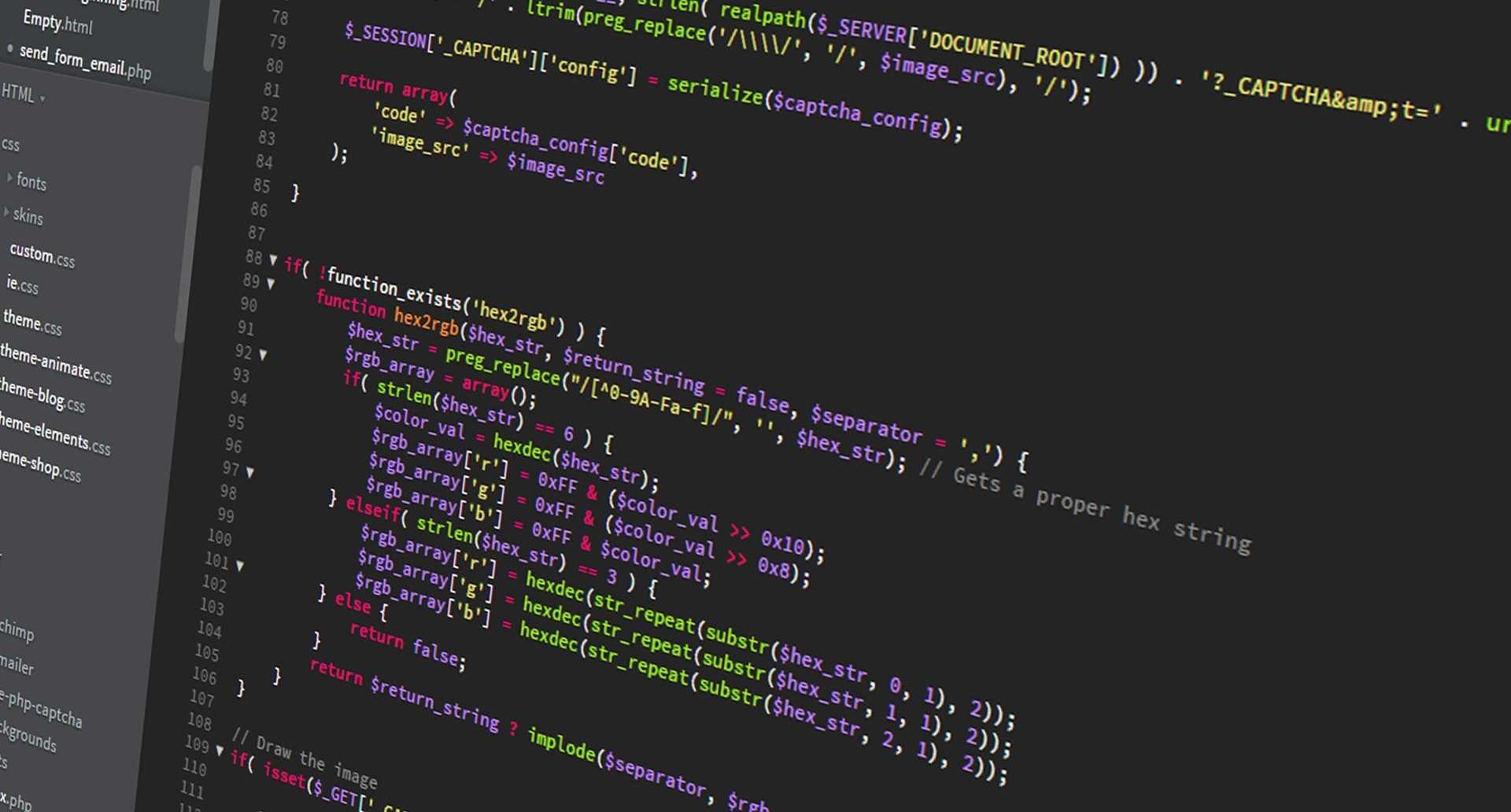
Object Oriented Programming with C# - Classes and Objects
1. Topics of discussion
In this tutorial, we are going to discuss what classes and objects are. So, let's have some fun.
2. Classes and objects explained
In the previous tutorial, we encountered the terms class and object for the first time. And it's time now to understand exactly what they are.
In OOP, a class is the abstract representation of a real-life concept, be it an object or a living being. What that means is that, in order to create a class, you would need to think about the core things that define the concept you are trying to represent in OOP. You need to think in terms of attributes, which define the properties of the object, and behavior, which define what the object can do.
In OOP, the properties of an object are stored in class attributes, which are properties defined at class level and are used to describe the real-life concept you have just represented. The behavior of the object is defined by creating methods inside that class.
Let's take a person as an example since we like to keep it original and not use something which is used in every other OOP course. What defines a person? Well, some might say the following things are common to a person:
- a first name and last name
- a date of birth
- a nationality
- a social security number
Of course, you could toss in other properties as well such as marital status, religion, eye color etc. But at the very essence, a person can be identified by those 5 properties.
Now that we understand what goes in a class, the next question we need to answer is: what is an object? Well, the answer is simple. An object is an instance of a class.
In order to make things even clearer, let's open the solution we created two tutorials ago in Visual Studio. Once that is done, right-click the project under Solution solution_name, select Add > New Folder and name it People. Now, right-click on that folder, select Add > New Item, select Class, and name it Person.cs. Once the file is created, place the following code in it:
public class Person { public string FirstName { get; set; } public string LastName { get; set; } public DateTime DateOfBirth { get; set; } public string Nationality { get; set; } public string SocialSecurityNumber { get; set; } public void SayHello() { Console.WriteLine($"Hi, my name is {FirstName} {LastName}"); } }
You will also need to add the following line at the beginning of the file, in order for your app to know where DateTime is located:
using System;
Right, so what we have here is basically a class called Person. Naming conventions in OOP state that class names must use Pascal Case when naming them, meaning that each word of the class must begin with a capital letter. In this class, we have added the properties we have mentioned above. Note the structure of each property line:
public data_type PropertyName {get; set; }
This is a shorthand notation that does the following things:
- creates a property called PropertyName which has a certain data_type
- creates a get method which allows you to access the value of the PropertyName property
- create a set method which allows you to change the value of the PropertyName property
Note that should you have omitted either the get or set method, then you would not have been able to access the value or change the value. We will discuss this in more detail when we talk about encapsulation.
As you can see, we have the attributes of a Person (which are the FirstName, LastName, and other variables) and a bit of behavior, represented by the SayHello() method, which basically prints out the name of the person.
So, now that we know what a class looks like, it's time to take a look at what an object looks like. You will have noticed that there is a Program.cs file in your project. Let's open that file and place the following code in it:
static void Main(string[] args) { Person person = new Person { FirstName = "Bruce", LastName = "Wayne" }; person.SayHello(); }
Note that the Person class is not available by default. In order to import it in the file, place your cursor at the end of the word Person, click Ctrl + . and select to import it.
And yeah, that's your first object right there. Note that C# also allows you to define the object as follows:
var person = new Person();
As you can see, we create instances of classes by using the new keyword. And in order to create objects, we use what is known as constructors.
That about covers it for this tutorial. In the next one, we are going to take an in-depth look at constructors. See you then.