Object Oriented Programming with C# - Constructors and Garbage Collection
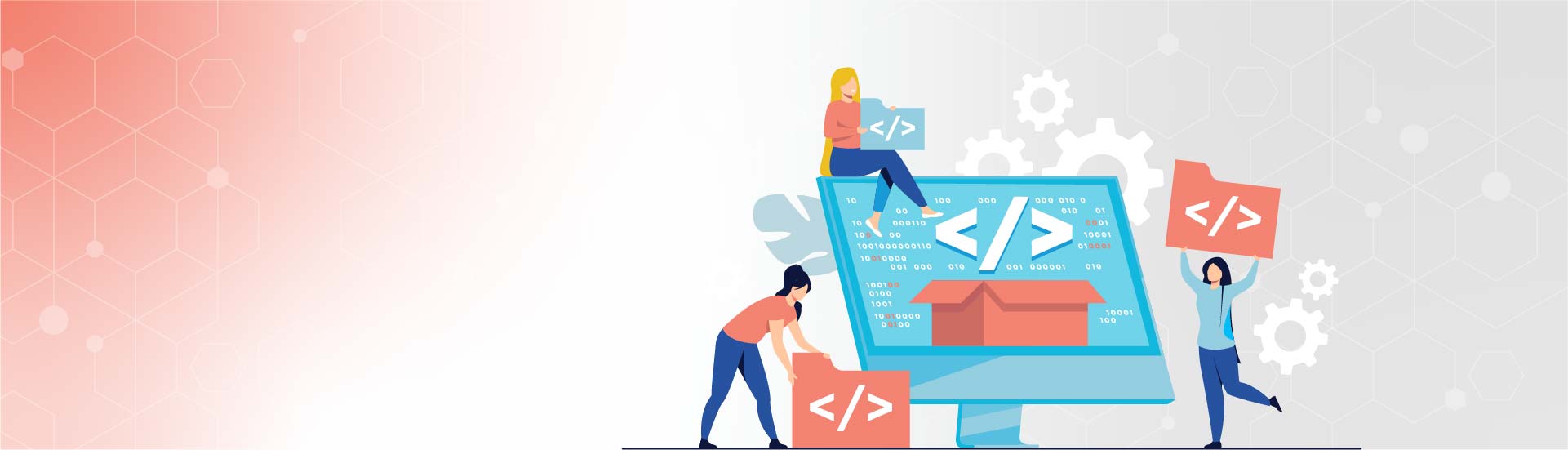
Object Oriented Programming with C# - Constructors and Garbage Collection
- Topics of discussion
- The construction and destruction of objects
1. Topics of discussion
In this tutorial, we are going to talk about constructors and how objects are destroyed. So, let's have some fun.
2. The construction and destruction of objects
In the previous tutorial, we instantiated an object using the new operator. In OOP, in order to instantiate a class, we use a thing called a constructor.
When it comes to constructors, you have two possibilities:
- using the implicit constructor; by default, each class has a constructor created for it, which accepts no parameters and instantiates each class property using its default value; numeric values are usually initialized with a value of 0, DateTime attributes are set to something like year 1, day 1, month 1 or something while string attributes are initialized as null
- using an explicit constructor, which can contain multiple extra instructions besides the basic initialization
In the previous tutorial, we used the implicit constructor, the one that C# generates for you. However, we can create explicit constructors as well. Let's modify the person class by adding an explicit constructor:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string Nationality { get; set; }
public string SocialSecurityNumber { get; set; }
public Person(string firstName, string lastName, DateTime dateOfBirth,
string nationality, string socialSecurityNumber)
{
FirstName = firstName;
LastName = lastName;
DateOfBirth = dateOfBirth;
Nationality = nationality;
SocialSecurityNumber = socialSecurityNumber;
}
public void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName}");
}
}
As you can see, a constructor is defined by using the class name, followed by a list of parameters inside some parentheses.
You can have as many constructors as you like with as many parameters as you like. Generally speaking though, the number of constructors is dependent on the purpose of the class. For example, when having a Person class, you could have a constructor that accepts parameters for all the class attributes and you can also have a parameterless constructor for easy instantiation.
Of course, now our code will not compile correctly, because once you define an explicit constructor, C# will no longer generate the implicit parameterless constructor. Let's fix the Program.cs file:
class Program
{
static void Main(string[] args)
{
Person person = new Person("Bruce", "Wayine", DateTime.Now, "American", "12345");
person.SayHello();
}
}
Now that we know how to create objects, it's time to figure out what happens to objects that are created and are no longer in use. While other programming languages would require you to invoke a destructor to destroy the object, C# does this automatically.
The gist of things is the following. Whenever you create a new object, it gets stored in either the heap memory or stack memory. We are not going to discuss the differences between those memory types, but the basic idea is that when you create an object, it gets stored in the memory. How long does it stay there? Well, until your program no longer needs it.
An object becomes obsolete in the following scenarios:
- it's no longer used in your program (meaning that you no longer invoke any methods from the class)
- you change the reference of the object (meaning you initialize the variable using the new operator again); in this case, the previously created value using the new operator becomes obsolete and can be deleted
Garbage collection is something that is out of scope for this tutorial. The thing that I want you to remember is that, once an object is created, C# takes care of destroying and freeing the memory used by said object when it is no longer used.
That about covers it for this tutorial. In the next one, we are going to talk about abstraction and encapsulation in C#. See you then.
Great news! We match words with deeds - and the truth is that... We can connect you with the top 1% developers in the world! Let's do some magic together! Contact us NOW!