Object-Oriented Programming With C# - Inheriting an Interface
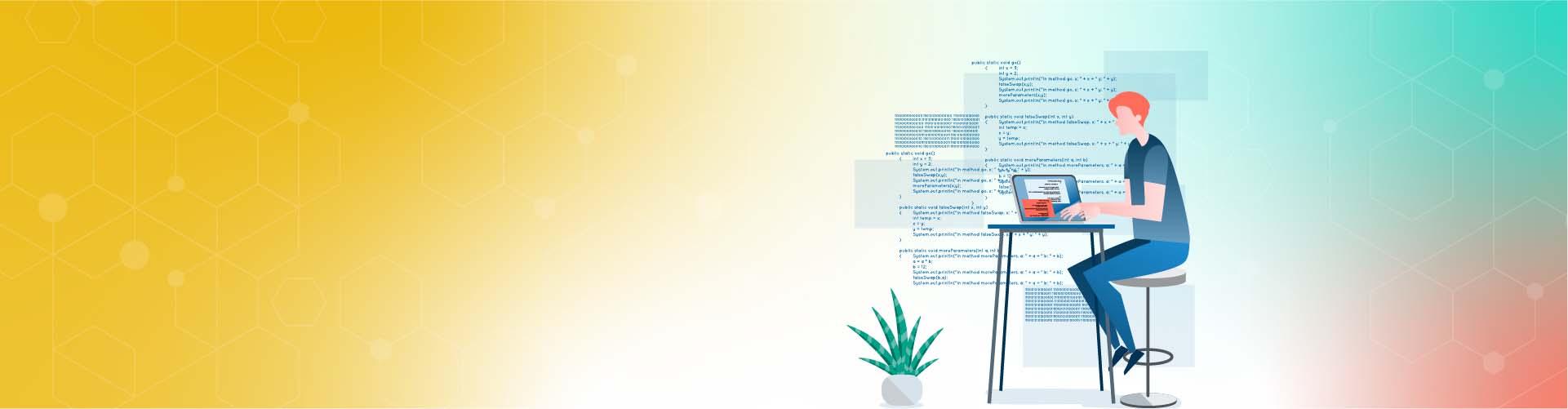
Object-Oriented Programming With C# - Inheriting an Interface
1. Topics of Discussion
2. Inheriting an Interface
1. Topics of Discussion
In this tutorial, we are going to talk about inheriting an interface. So, let's have some fun.
2. Inheriting an Interface
In C#, an interface is defined by using the interface keyword. Shocking, right? As for its purpose, an interface usually consists of some methods which are defined but not implemented. In other words, an interface contains a bunch of method signatures with their corresponding return type.
The idea behind interfaces is to allow for different classes to have different implementations of those methods. The caveat here is that, unlike classes, you must provide an implementation for all the methods of an interface when inheriting (or implementing) it.
Let's create an interface called IEmployable. Right-click the People folder (or any other folder in your project), click Add > New Item... and select Interface. Name it IEmployable and put the following code in it:
public interface IEmployable
{
void DisplayEmploymentStatus();
}
And now, let's make the Student class implement it:
public class Student : Person, IEmployable
{
public Student(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber) : base(firstName, lastName, dateOfBirth, nationality, socialSecurityNumber)
{
}
public string College { get; set; }
public bool HasGraduated { get; set; }
public new void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName} and I am a student at {College}");
}
public void DisplayEmploymentStatus()
{
Console.WriteLine("Employment during college is hard...");
}
}
Notice that we have added the IEmployable interface next to the Person class. If you add the interface without overriding the method or methods from it, a compilation error will occur. As you can see, we have provided an implementation for the method so our code would run.
As you can see, we did not provide any implementation for the method in the interface. Note that we didn't add the public keyword to the DisplayEmploymentStatus method in the IEmployable interface.
The keyword is unnecessary because of the following two aspects:
- methods in an interface are public methods by definition
- methods in an interface are abstract methods by definition (we'll discuss this in more detail in the next tutorial)
Before we leave, here are two things that I want you to remember:
- an interface can inherit another interface; this may impact the number of methods you have to override; so, if InterfaceB inherits InterfaceA and ClassC implements InterfaceB, then ClassC must override the methods defined in both InterfaceA and InterfaceB
- unlike class inheritance, you can implement as many interfaces as you want
And that about covers it for this tutorial. In the next one, we are going to talk about abstract classes and methods and how one can prevent inheritance. See you then.
References
Upstack gives you the ability to search for the perfect software programmer for your project by skill, location, experience level and more. Contact us!