Object Oriented Programming with C# - OOP Principles
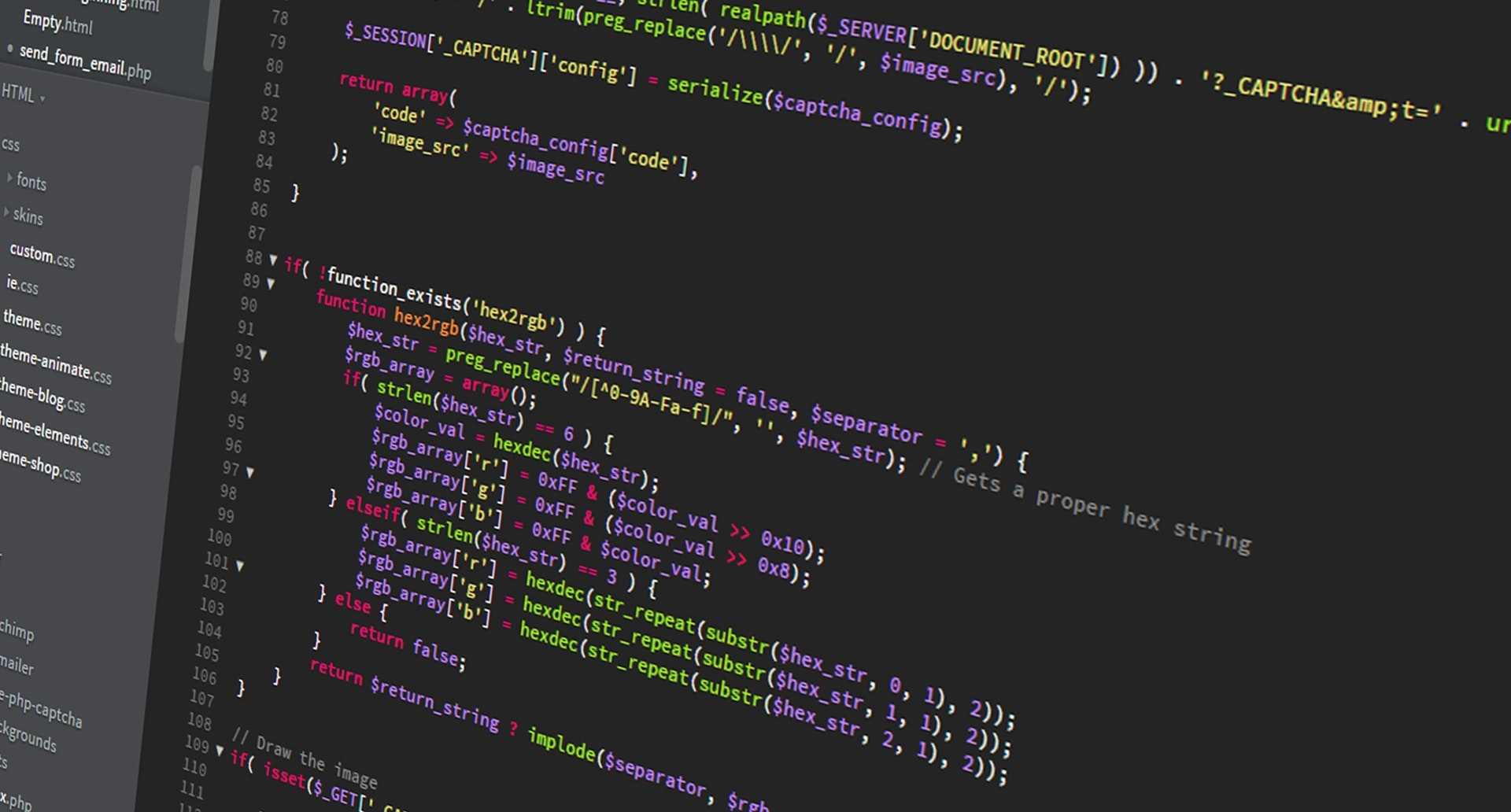
Object Oriented Programming with C# - OOP Principles
Topics of discussion
In this tutorial, we are going to talk about the 4 principles of OOP. So, let's have some fun.
2. The 4 OOP principles
When it comes down to OOP, there are 4 principles on which this paradigm is built on. And in this tutorial, we are going to go through all of them.
The first principle is called abstraction. This concept is about as abstract in definition as it is in name. In the next tutorial, we are going to discuss classes and objects in more detail, but for now, we need to understand that OOP uses a class to define an abstraction of a real-life object. And the abstraction principle refers to the idea that the classes you create should not be dependent on an instance of that class (which is what an object is, as we'll learn next time). Basically, a class should be able to stand on its own without having to rely on any information for any of its instances.
The second principle is called encapsulation. This principle refers to the idea that class attributes should not be directly accessible in your program. Rather, the process of accessing a variable should be done via what is known as accessor methods or getter methods.
The third principle is called polymorphism. This principle refers to the idea that you can have methods that have the same name but produce different results. As we'll learn in future tutorials, polymorphism can be done in two ways:
- changing the method signature (the name of the method remains unchanged)
- changing what the method does in a subclass (which can occur when using inheritance, which just so happens to be the final principle)
Lastly, the fourth principle of OOP is called inheritance. This principle refers to the idea that a class can inherit certain properties and methods from another class while also allowing for the child class to get enhanced with other specific attributes and methods. A child class can also override the functionality of methods it inherits from its parent class. In C#, inheritance can be done in two ways:
- you can inherit from another class
- you can inherit from an interface
We'll discuss these in a future tutorial as well, so don't worry about it.
That about covers it for this tutorial. In the next one, we are going to talk about classes and objects. See you then.