Object-Oriented Programming With C# - Inheriting A Class In C#
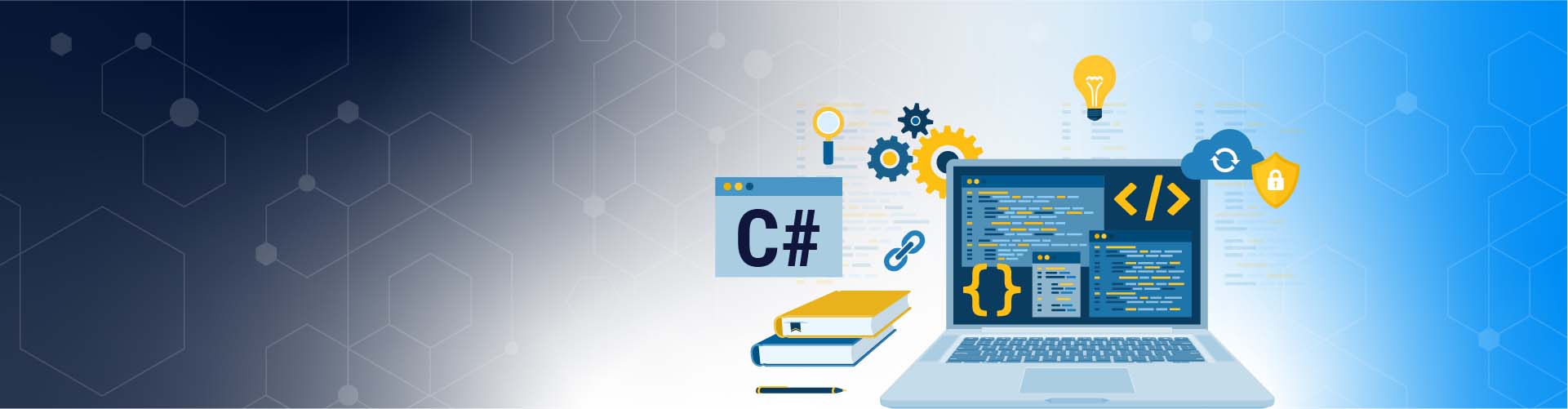
Object-Oriented Programming With C# - Inheriting A Class In C#
- Topics of Discussion
- Class Inheritance
- Polymorphism with Method Overriding
1. Topics of Discussion
In this tutorial, we are going to talk about how we can inherit a class in C#, while also learning about polymorphism with method overriding. So, let's have some fun.
2. Class Inheritance
Inheritance is the fourth and final OOP concept we will discuss. It basically allows a class to enhance its own functionality by inheriting the functionality already defined in another class. This helps with what is known as code reusability since you don't have to rewrite your code to get your class to work. There are two types of inheritance in C#: you can inherit a class or an interface. Interface inheritance will be discussed in the next tutorial.
When it comes to class inheritance, you can only inherit from one class. There is no multiple class inheritance and for good reason. The reason is that, should you inherit from two classes that define a method with the same name, then C# would be a bit confused as to what method you are trying to call in a child class.
Anyhow, let's create a class called Student which will inherit from Person. Create the class inside the People folder and place the following code inside it:
public class Student : Person
{
public string College { get; set; }
public bool HasGraduated { get; set; }
}
And let's review the Person class as well:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string Nationality { get; set; }
public string SocialSecurityNumber { get; set; }
public Person() { }
public Person(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber)
{
FirstName = firstName;
LastName = lastName;
DateOfBirth = dateOfBirth;
Nationality = nationality;
SocialSecurityNumber = socialSecurityNumber;
}
public void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName}");
}
}
As you can see, inheriting from a class is quite easy. Now, let's talk about some inheritance concepts which you should remember:
- as stated, you can only inherit from one class
- when a class inherits from another, the class which inherits is called a child class and the class which gets inherited is called the parent class or base class
- when a class inherits another, it gains access to the public and protected properties and methods from the base class
- if the child class inherits a class that itself is a child of another class, then the first child will gain access to the public and protected properties from both classes
- constructor wise, remember that the base class constructor will get invoked before the child class constructor
- because of the last bullet point, if a base class defines an explicit constructor which has a parameter, then you have two options: the child class must define an explicit constructor of its own, which must invoke the base class constructor
you can define a parameterless constructor in the base class, which will get invoked automatically in the child class constructor - aside from constructors, you can also invoke base class methods
In the example above, we have defined a parameterless constructor in the base class, which solves our issues regarding constructors. Let's modify the code to see how we can handle base classes that define explicit constructors with parameters without defining a parameterless one:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string Nationality { get; set; }
public string SocialSecurityNumber { get; set; }
public Person(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber)
{
FirstName = firstName;
LastName = lastName;
DateOfBirth = dateOfBirth;
Nationality = nationality;
SocialSecurityNumber = socialSecurityNumber;
}
public void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName}");
}
}
To make our code work, we need to modify the Student class as follows:
public class Student : Person
{
public Student(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber) : base(firstName, lastName, dateOfBirth, nationality, socialSecurityNumber)
{ }
public string College { get; set; }
public bool HasGraduated { get; set; }
}
Notice how we invoke the base constructor by using the base keyword. You can use the base keyword to invoke a base class method in the child class like this:
base.MethodName();
3. Polymorphism With Method Overriding
When inheriting from a class, it is possible to change the functionality of a base class method in the child class. This process is called method overriding and is the second type of polymorphism that can be implemented in C#. Let's override the SayHello( ) method in the Student class:
public class Student : Person
{
public Student(string firstName, string lastName, DateTime dateOfBirth, string nationality, string socialSecurityNumber) : base(firstName, lastName, dateOfBirth, nationality, socialSecurityNumber)
{
}
public string College { get; set; }
public bool HasGraduated { get; set; }
public new void SayHello()
{
Console.WriteLine($"Hi, my name is {FirstName} {LastName} and I am a student at {College}");
}
}
Let's test this out, shall we? Place the following code in the Program.cs file:
class Program
{
static void Main(string[] args)
{
Person person = new Person("Bruce", "Wayne", DateTime.Now, "American", "12345");
person.SayHello();
var student = new Student("Damian", "Wayne", DateTime.Now, "American", "12345");
student.College = "Batman School of Heroes";
student.SayHello();
}
}
And that about covers it for this tutorial. In the next one, we are going to discuss inheriting an interface. See you then.
References
Connect with Upstack for hiring pre-vetted tech talent for your project! Let’s grow together!