Ruby CGI Scripts
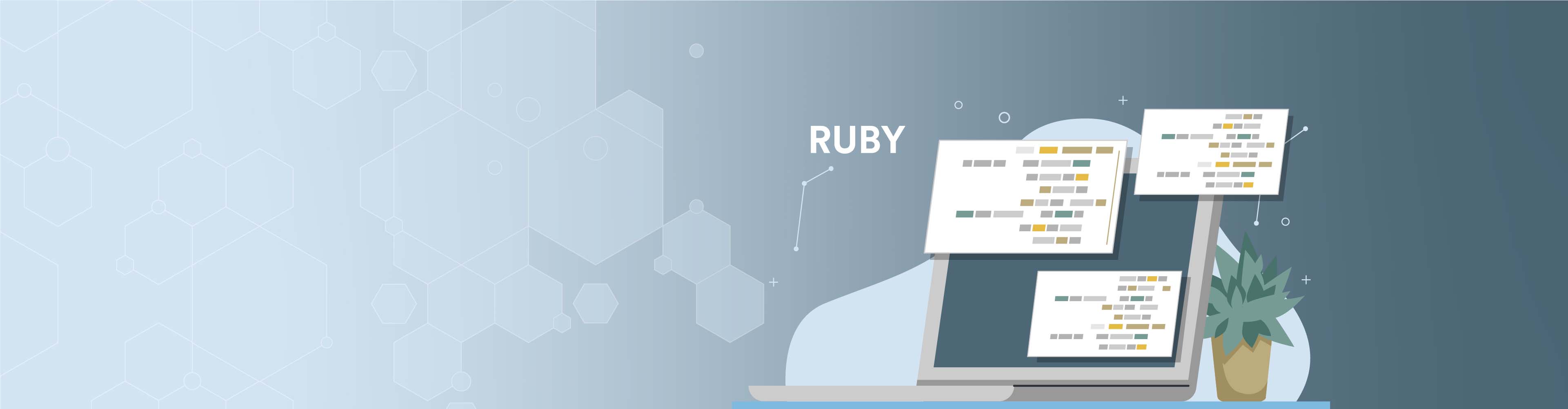
Ruby CGI Scripts
Ruby is a programming language with wide use; it does not only work in web development language; however, Ruby development is also common in web applications and web tools. In addition to using Ruby to write your own SMTP server, FTP program, or Ruby web server, you can also use Ruby CGI programming.
Configuration and support of Webserver
Before you go ahead to use CGI programming, ensure your web server has been configured to work with CGI and CGI handlers.
Apache supports CGI configuration:
Set up the CGI directory:
ScriptAlias /cgi-bin/ /var/www/cgi-bin/
The HTTP server for the execution of CGI programs is saved in a pre-configured directory. The name of the directory is the CGI directory and conventionally called /var/www/CGI-bin directory.
The file extension for CGI is .cgi, and ruby extension (.rb) can also be used.
The default Linux server configuration to run the cgi-bin directory is /var/www
If you want to run the CGI scripts with another directory, you can change the configuration file (httpd.conf) as follows:
<Directory "/var/www/cgi-bin">
AllowOverride None
Options +ExecCGI
Order allow,deny
Allow from all
</Directory>
Add .rb suffix AddHandler, so that we can access the .rb end of the Ruby script file:
AddHandler cgi-script .cgi .pl .rb
Writing CGI Scripts
Basically, Ruby CGI scripts will look like the following:
#!/usr/bin/ruby
puts "HTTP/1.0 200 OK"
puts "Content-type: text/html\n\n"
puts "<html><body>This is a test</body></html>"
If you call the test.cgi script and uploaded it to a web hosting provider based on Unix with the right permissions; you can utilize it as a CGI script.
For instance, if your site https://www.upstack.com/ is hosted by a Linux web hosting provider and you upload test.cgi in your home directory and give execution permission, go to https://www. Example.com/test.cgi should return an HTML page, indicating that it is a test.
When a Web browser requests the test.cgi, the webserver searches for test.cgi on the website and executes through the Ruby Interpreter. The Ruby script sends a simple HTTP header and returns an HTML document.
Using CGI.RB
Ruby features a special library known as CGI, and it enables a more advanced interaction greater than the preceding CGI script.
With the cgi, you can create a simple CGI script:
#!/usr/bin/ruby
require 'cgi'
cgi = CGI.new
puts cgi.header
puts "<html><body>This is a test</body></html>"
Form Processing
There are two ways to access HTML query parameters using CGI classes. Suppose we have URL like /cgi-bin/test.cgi?FirstName = Emmanuel&LastName = Mario.
When you use CGI#[] directly as illustrated below, you will have access to the FirstName and LastName parameters.
#!/usr/bin/ruby
require 'cgi'
cgi = CGI.new
cgi['FirstName'] # =>
["Emmanuel"]
cgi['LastName'] # => ["Mario"]
You can also access the form variables using another way. This code will provide the hash for all keys and values:
#!/usr/bin/ruby
require 'cgi'
cgi = CGI.new
h = cgi.params # => {"FirstName"=>["Emmanuel"],"LastName"=>["Mario"]}
h['FirstName'] # => ["Emmanuel"]
h['LastName'] # => ["Mario"]
You can find below the code to use for retrieving all keys:
#!/usr/bin/ruby
require 'cgi'
cgi = CGI.new
cgi.keys # => ["FirstName", "LastName"]
If there are multiple fields in a form with the same name, the corresponding values are returned to the script will in the form of an array. The [] accessor only returns the result of the first such .index params method to get them.
In the code illustration below, as an assumption, the form contains three fields known as “name” and we input three different names “Maria” “Jude” “Jason”:
#!/usr/bin/ruby
require 'cgi'
cgi = CGI.new
cgi['name'] # => "Maria"
cgi.params['name'] # => ["Maria", "Jude", "Jason"]
cgi.keys # => ["name"]
cgi.params # => {"name"=>["Maria", "Jude", "Jason"]}
Ruby will automatically handle the GET and POST methods. And the treatment for the two methods is the same and not in any way separate.
A basic and associated data capable of sending the correct data will have the HTML code like the following:
<html>
<body>
<form method = "POST" action = "http://www.example.com/test.cgi">
First Name :<input type = "text" name = "FirstName" value = "" />
<br />
Last Name :<input type = "text" name = "LastName" value = "" />
<input type = "submit" value = "Submit Data" />
</form>
</body>
</html>
Creating Forms and HTML Form
There are many ways to create HTML in CGI, and each HTML tag contains a corresponding method. Prior to using these methods, the report must be CGI to create a CGI.new object. To facilitate the label nesting, these methods are included as blocks of code; code blocks return strings as a content tag. Following is an example:
#!/usr/bin/ruby
require "cgi"
cgi = CGI.new("html4")
cgi.out{
cgi.html{
cgi.head{ "\n"+cgi.title{"This Is a Test"} } +
cgi.body{ "\n"+
cgi.form{"\n"+
cgi.hr +
cgi.h1 { "A Form: " } + "\n"+
cgi.textarea("get_text") +"\n"+
cgi.br +
cgi.submit
}
}
}
}
String escape
While working with parameters in the URL or HTML form data, it is necessary that you specify special characters for the escape; some of them are quotation marks ( "), slash (/).
In the Ruby CGI object, you will be given CGI.escape CGI.unescape and methods to handle escaping these characters:
#!/usr/bin/ruby
require 'cgi'
puts CGI.escape(Emmanuel Mario/ Serious & Intelligent")
The execution of the above code is as follows:
#!/usr/bin/ruby
require 'cgi'
puts CGI.escape(Emmanuel Mario/ Serious & Intelligent")
Some examples include:
#!/usr/bin/ruby
require 'cgi'
puts CGI.escapeHTML('<h1>Emmanuel Mario/Serious & Intelligent</h1>')
The execution of the above code is as follows:
<h1>Emmanuel Mario/ Serious & Intelligent</h1>'
Some CGI methods
- CGI::new([level=”query”])
- CGI::escape (str)
- CGI::unescaped (str)
- CGI::escapeHTML (str)
- CGI::unescapeHTML (str)
- CGI::escapeElement( str[,element…])
- CGI::unescapeElement( str[,element…])
- CGI::parse(query)
- CGI::pretty(string[, leader=””])
- CGI::rfc1123_date(time)
At Upstack we help you grow your business faster. We connect you with the top 1% developers in the world. Contact us NOW!