Ruby Code Examples
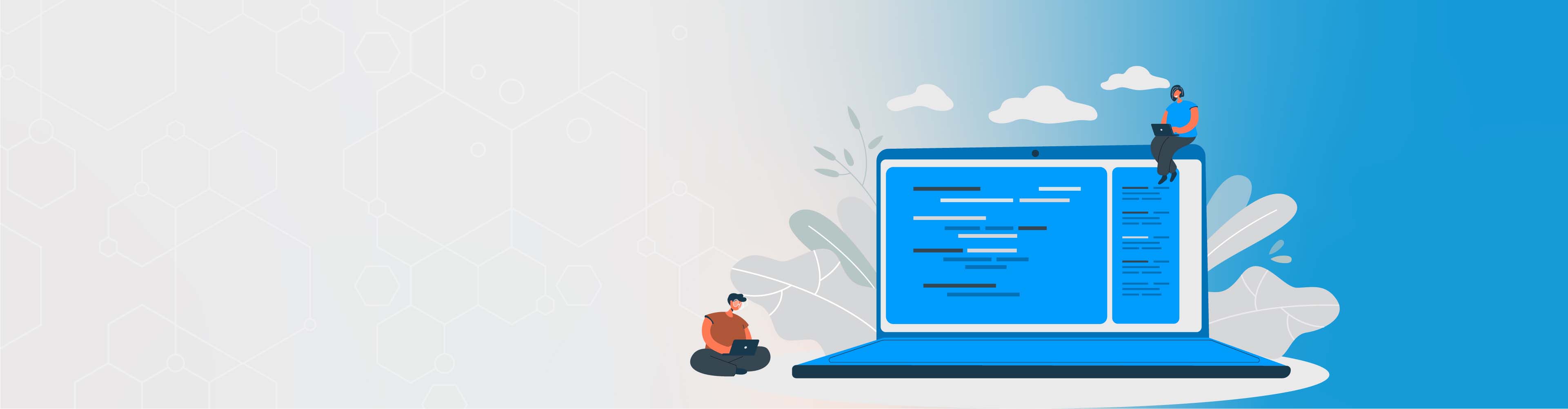
Ruby Code Examples
Ruby is a basic scripting language for use in front and back-end web development and similar applications. It is a strong, object-oriented language. Furthermore, its syntax is advanced and easy to understand, and many consider it as easy as coding using English.
Printing Hello World! In Ruby
If you want to print Hello World! Or any other words, the following are the two methods you can use
- puts: Which signifies the put strings. After the string, there will be an addition of a new line.
- print: This function is similar to put; however, there won't be an implicit addition of newlines like input
Ruby code to print "Hello World!"
=begin
Ruby program to print Hello World.
=end
puts "Hello World!"
print "Hello World!"
puts "Hello World!"
Output:
Hello World!
Hello World! Hello World!
Addition of two Integers using the Ruby program
Adding two numbers in Ruby
If you are given two integer numbers in Ruby, and you need to add them together. The problem might look straightforward; however, it demands you use some methods. If you want to know the correct value of the string, you will need to add the values together.
Methods used:
- puts: It helps users to view some messages
- gets: This function allows the taking of the user’s input
- .to_i: When we get inputs using the gets method, it's a string. For more calculations, it is imperative to convert it to an integer to get accurate results, and this method returns the conversion of the given string.
- +: This accepts two numerical parameters and also returning a numeric value
=begin
Ruby program to add two numbers.
=end
# input the numbers and converting
# them into an integer
puts "Enter first value: "
num1=gets.chomp.to_i
puts "Enter second value: "
num2=gets.chomp.to_i
# finding sum
sum=num1+num2
# printing the result
puts "The sum is #{sum}."
Output:
First, run:
Enter first value:
123
Enter second value:
456
The sum is 579
Second run:
Enter first value:
-120
Enter second value:
20
The sum is -100
Strings
There are different ways to create strings in Ruby, and it’s a typical variation of how Perl can create strings. Double quotes allow the interpolation of values into the string while it is not possible with single quotes. Many escape characters are enclosed in single quotes, \n is treated as double characters, then a backward slash and an n
# You can substitute variables with Double-quoted strings.
a = 17
print "a = #{a}\n";
print 'a = #{a}\n';
print "\n";
# If you're verbose, you can create a multi-line string like this.
b = <<ENDER
This is a long string; some instructions or agreements can be found here.
a = #{a}.
ENDER
print "\n[[[" + b + "]]]\n";
print "\nActually, any string
can span lines. The line\nbreaks just become
part of the string.
"
print %Q=\nThe highly intuitive "%Q" prefix allows alternative delimiters.\n=
print %Q[Bracket symbols match their mates, not themselves.\n]
String operations
s = "Good morning.How are you?"
print s.length, " [" + s + "]\n"
# Selecting a character in a string gives an integer ascii code.
print s[4], "\n"
printf("%c\n", s[4])
# The [n,l] substring gives the starting position and length. The [n..m]
# form gives a range of positions, inclusive.
print "[" + s[4,4] + "] [" + s[6..15] + "]\n"
print "Wow " * 3, "\n"
print s.index("there"), " ", s.index("How"), " ", s.index("bogus"), "\n"
print s.reverse, "\n"
a = [ 45, 3, 19, 8 ]
b = [ 'sam', 'max', 56, 98.9, 3, 10, 'jill' ]
print (a + b).join(' '), "\n"
print a[2], " ", b[4], " ", b[-2], "\n"
print a.sort.join(' '), "\n"
a << 57 << 9 << 'phil'
print "A: ", a.join(' '), "\n"
Ruby Arrays
Ruby arrays have the same operation as the strings. The same way a quote notation makes a String object, a bracket notation also makes an Array object.
b << ‘Brian’ << 48 << 220
print "B: ", b.join(' '), "\n"
print "pop: ", b.pop, "\n"
print "shift: ", b.shift, "\n"
print "C: ", b.join(' '), "\n"
b.delete_at(2)
b.delete('alex')
print "D: ", b.join(' '), "\n"
Ruby code to find the area of the rectangle
=begin
Ruby program to find Area of Rectangle.
=end
# input length and breadth, and
# convert them to float value
puts "Enter length:"
l=gets.chomp.to_f
puts "Enter width:"
w=gets.chomp.to_f
# calculating area
area=l*w
# printing the result
puts "Area of Rectangle is #{area}"
Output
4
First run:
Enter length:
12.3
Enter width:
5
Area of Rectangle is 61.5
Second run:
Enter length:
1.234
Enter width:
0.78
Area of Rectangle is 0.96252
Ruby code to calculate the sum of all even numbers
Method 1: Using the classical way using %2
=begin
Calculating the sum of all even numbers up to n using the Ruby program.
=end
sum=0
puts "Enter n:-"
n=gets.chomp.to_i
i=1
while(i<=n)
if(i%2==0)#using % operator
sum=sum+i
i=i+1
else
i=i+1
end
end
puts "The sum is #{sum}"
Output:
RUN 1:
Enter n:-
5
The sum is 6
RUN 2:
Enter n:-
60
The sum is 930
Ruby Classes
# Class names must be in Capitals. Technically, it's a constant.
class UPSTACK
# The initialize method is the constructor. The @val is
# an object value.
def initialize(v)
@val = v
end
# Set it and get it.
def set(v)
@val = v
end
def get
return @val
end
end
# Objects are created with the new method of the class object.
a = Upstack.new(10)
b = Upstack.new(22)
print "A: ", a.get, " ", b.get,"\n";
b.set(34)
print "B: ", a.get, " ", b.get,"\n";
# Most times, Ruby classes are unfinished. This does not
# change Upstack, it only adds to it.
class Fred
def inc
@val += 1
end
end
a.inc
b.inc
print "C: ", a.get, " ", b.get,"\n";
# Objects may have methods all to themselves.
def b.dec
@val -= 1
end
begin
b.dec
a.dec
rescue StandardError => msg
print "Error: ", msg, "\n"
end
print "D: ", a.get, " ", b.get,"\n";
We are growth pros. CONTACT US so we can help you scale your team with top 1% talent worldwide!