Ruby Hash Examples
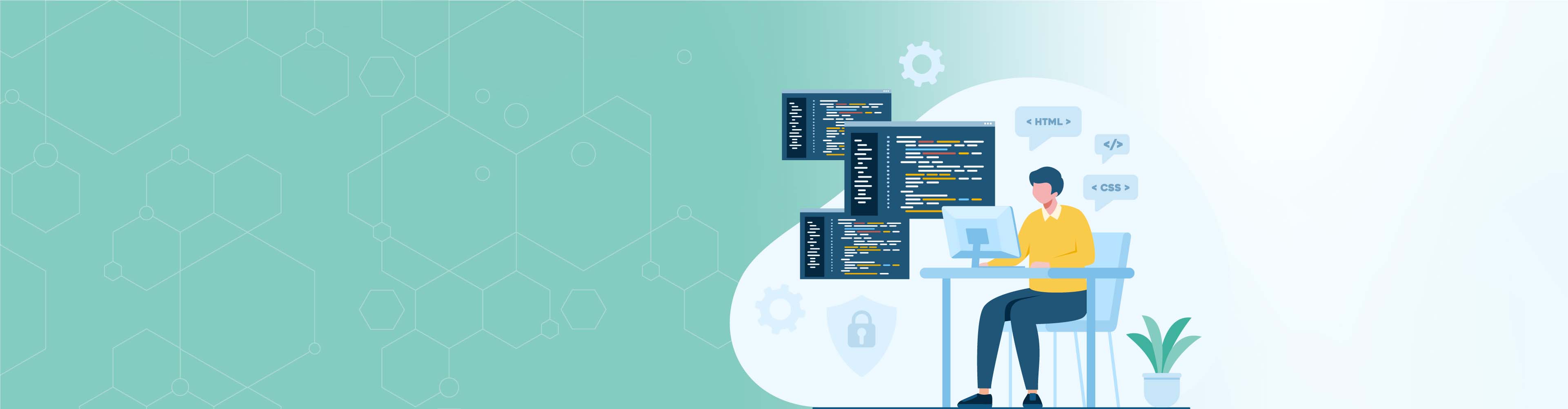
Ruby Hash Examples
A hash is a data structure like an array; however stores items according to assigned keys. This is in contrast to arrays storing items using an ordered index. Hash entries are often called key/value pairs. This will create an associative data representation. The most common is to use symbols as keys and any type of data as values to create a hash. Each key-value pair in the hash is enclosed in braces {} and is separated by commas.
You can create Hashes using two syntaxes. The older syntax uses a => sign for separating the key and value.
irb :001 > old_syntax_hash = {:name => 'mark'}
=> {:name=>'mark'}
The newer syntax is introduced in Ruby version 1.9 and is much simpler. As you can see, the result is the same.
irb :002 > new_hash = {name: 'mark'}
=> {:name=>'mark'}
Create a Hash using Key/Value Pairs
Hashes are used for storing key/value pairs. The key/value pair feature an identifier to identify the hash variable you want to access and a variable to be stored in the hash position. For example, teachers can save the grades of students in a hash. Mark’s grade will be accessed in a hash using the key “Mark,” and the variable that will be stored at that position is Mark’s grade.
You can create a hash variable the same way as an array variable. The easiest way to do this is to create an empty hash object and populate it with key/value pairs. Notably, the index operator is used; however, the student’s name is used as a replacement of a number.
Keep in mind that hashes are not ordered, which means that there is no beginning or end defined as in an array. Therefore, you cannot add to the hash. Use the index operator to simply insert the value into the hash.
#!/usr/bin/env ruby
grades = Hash.new
grades["Mark"] = 82
grades["John"] = 94
grades["Cindy"] = 58
puts grades["John"]
Hash Literals
As with arrays, hash literals can be used to create hashes. Hash literals use curly braces as a replacement for square brackets, and key-value pairs are connected by =>. For instance, a hash with a single key/value for pair Mark / 84 would be: {"Mark" => 84}. Other keys/value pairs can be added to the literal hash and separated with commas. Below is an example of a hash created for students with their grades
#!/usr/bin/env ruby
grades = { "Mark" => 82,
"John" => 94,
"Cindy" => 58
}
puts grades["John"]
Fetching keys in hash
Fetching the keys of a hash in an array requires using the key method on a hash object.
> my_hash = {key1:"value1", key2: "value2", key3: "value3"}
=> {:key1=>"value1", :key2=>"value2",:key3=>"value3"}
## To retreive all the keys
> my_hash.keys
=> [:key1, :key2, :key3]
Removing all key-value pairs from a hash
If you want to remove the key-value pair from the hash, you will need to use the clear method:
> my_hash = {key1: "value1", key2: "value2", key3: "value3"}
=> {:key1=>"value1", :key2=>"value2", :key3=>"value3"}
> my_hash.clear
=> {}
> my_hash
=> {}
Deleting the key-value pair from the hash:
If you are deleting the key-value pairs from the hash by using keys or specifying a particular condition using the delete method.
> my_hash = {key1: 2, key2: 3, key3: 4, key4: 5, key6: 6}
=> {:key1=>2, :key2=>3, :key3=>4, :key4=>5, :key6=>6}
## To delete the key2 key-value pair:
> my_hash.delete(:key1)
=> 2
## Now check my_hash the key1 key-value pair has been removed from my_hash
> my_hash
=> {:key2=>3, :key3=>4, :key4=>5, :key6=>6}
## To delete all the even number delete_if can be used liked this
> my_hash = {key1: 2, key2: 3, key3: 4, key4: 5, key6: 6}
=> {:key1=>2, :key2=>3, :key3=>4, :key4=>5, :key6=>6}
> my_hash.delete_if { |key,value| value%2==0 }
=> {:key2=>3, :key4=>5}
Iterating over hash
There are several ways of iterating over a hash.
## Iterating over hash by using key-value pair as a parameter for the block
> my_hash = {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
> my_hash.each{|key, value| puts "Key is : #{key} Value is : #{value}" }
Key is : 1 Value is : 15
Key is : 2 Value is : 30
Key is : 3 Value is : 45
Key is : 4 Value is : 60
Key is : 5 Value is : 75
=> {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
## Iterating over hash by using only key as a parameter for the block
> my_hash.each_key { |key| puts "Key is #{key}" }
Key is 1
Key is 2
Key is 3
Key is 4
Key is 5
=> {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
## Iterating over hash by using only value as a parameter for the block
> my_hash.each_value { |value| puts "Value is #{value}" }
Value is 15
Value is 30
Value is 45
Value is 60
Value is 75
=> {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
Merging two hashes
If we have two hashes and want to create a new hash featuring all the keys and values of the two hashes. We can achieve that using the merge method.
> my_hash = {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
=> {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
> my_hash2 = {"6" => 90, "7" => 105}
=> {"6"=>60, "7"=>70}
my_hash.merge(my_hash2)
=> {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75, "6"=>90, "7"=>105}
Checking the presence of a key in a hash
There are several ways to return true or false to determine if the hash contains a specific key or not:
> my_hash = {"1"=>15, "2"=>30, "3"=>45, "4"=>60, "5"=>75}
## Using has_key? method
> my_hash.has_key?("1")
=> true
> my_hash.has_key?("6")
=> false
## Using include? method
> my_hash.include?("1")
=> true
## Using key? method
> my_hash.key?("1")
=> true
## Using member? method
> my_hash.member?("1")
=> true
## Test whether hash contains some value
> my_hash.value?(15)
=> true
Many companies face challenges with finding top tech talent, from candidate qualifications to team dynamics, to economics that fit their financial scale. Our unique solution for hiring top 1% independent contractors worldwide addresses all of these concerns! Get in touch!