How To Compare DateTime In JavaScript
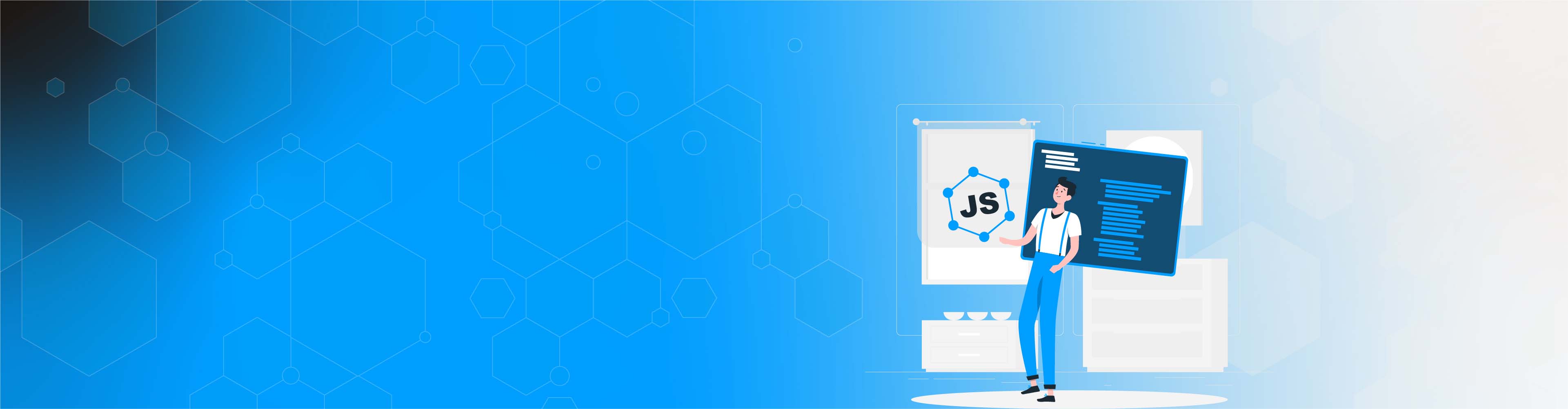
How To Compare DateTime In JavaScript
Date and time are an indispensable part of our daily lives, so they occupy an important position in computer programming. It may be necessary to create a calendar on a website, schedule, or interface to set appointments in JavaScript. These apps need to display the relevant time according to the current time zone of the user or calculate the arrival and departure time or the start and end time. In addition, you may need to use JavaScript to run reports on a particular day.
A JavaScript date is an embedded object that internally stores the number of milliseconds from January 1, 1970, and externally, it is presented as a highly formatted, environmentally dependent wire. In this article, I will teach you how to compare dates and times.
JavaScript Date Input
Generally, there are three types of input formats for JavaScript date:
- ISO Date 2021-03-20; The International Standard
- Short Date 03/20/2021
- Long Date Mar 20, 2021, or 20 Mar 2021
JavaScript Date Output
Without the input format, the standard output dates for JavaScript in a complete string format is:
SAT Mar 20 2021 17:00:00 GMT-0700 (Pacific Daylight Time)
JavaScript ISO Dates
ISO 8601 is the international standard for date and time representation.
The ISO 8601 syntax (YYYY-MM-DD) is also the most used JavaScript data format:
Example (Complete date)
const d = new Date("2021-03-20");
Ways to Create a JavaScript Date Object
JavaScript date object is used to create dates and times.
Below are different ways to create a date object in JavaScript:
- New Date(); for example today = new Date().
- New Date(milliseconds); for example inauguration_day = new Date("March 16, 2021 12:05:00")
- New Date(dateString); for example inauguration_day = new Date(21,8,15).
- New Date(year, month, day, hours, minutes, seconds, milliseconds); for example inauguration_day = new Date(21,11,14,20,08,0).
How to Compare Date and Time in JavaScript?
Comparing Two Dates and Time in JavaScript
In the code example below, we created two new dates with varying times
// Compare two Dates with Time in Javascript
function CompareDate() {
// create new date using Date Object => new Date (Year, Month, Date, Hr, Min, Sec);
var firstOne = new Date (2021, 10, 25, 14, 55, 59);
var secondTwo = new Date (2021, 10, 25, 12, 10, 20);
//Note: 10 is month i.e. Oct
if (firstOne > secondTwo) {
alert("First Date is greater than Second Date.");
} else {
alert("Second Two is greater than the First Date.");
}
}
CompareDate();
Comparing Past and Future Date with a Date
In the example below, we created two dates while comparing them
//Compare Two Dates in Javascript
function CompareDate() {
//Note: 00 is month i.e. January
var firstDate = new Date(2021, 4, 15); //Year, Month, Date
var secondDate = new Date(2022, 4, 15); //Year, Month, Date
if (firstDate > secondDate) {
alert("First Date is greater than Second Date.");
} else {
alert("Second Date is greater than the First Date.");
}
}
CompareDate();
Comparing the same dates with different timings
<script>
var d1=new Date("2020-03-10, 10:10:10"); //yyyy-mm-dd hh:mm:ss
var d2=new Date("2020-03-10, 10:10:50"); //yyyy-mm-dd hh:mm:ss
if(d1>d2)
{
document.write("False, d1 & d2 dates are same but d2 time is greater than d1 time");
}
else if(d1<d2)
{
document.write("True, d2 time is greater than d1 time.");
}
else
{
Document. Write ("The date and time are same and equal");
}
</script>
Comparing date with getTime()
An excellent approach to compare dates is using the getTime() function. This function allows you to convert dates to numeric values for direct comparison.
Example: Comparing current date and time with a given date and time.
<script>
var d1=new Date("2020-10-10, 10:10:10"); //yyyy-mm-dd hh:mm:ss
var currentdate=new Date(); //fetch the current date value
if(d1.getTime()<currentdate.getTime())
{
document.write("True, currentdate and time are greater than d1");
}
else if(d1.getTime()>currentdate.getTime())
{
document.write("False");
}
else
{
document.write("True, equal");
}
</script>
Compare Today's Date with Any Other Date in JavaScript.
In this example, two dates are created, the current date and/or any other date, and compare these two dates.
// Compare Today's date with another Date
function CompareDate() {
var todayDate = new Date(); //Today Date
var anyDate = new Date(2021, 11, 25);
if (todayDate > anyDate) {
alert("Today's Date is greater than other given Date.");
} else {
alert("Today's Date is smaller than other given Date.");
}
}
CompareDate();
Compare Past and future dates with a given date.
In this example, we create two dates and also did the dates comparison.
//Compare Two Dates in JavaScript
function CompareDate() {
//Note: 00 is month i.e. January
var firstDate = new Date(2019, 3, 15); //Year, Month, Date
var secondDate = new Date(2020, 3, 15); //Year, Month, Date
if (firstDate > secondDate) {
alert("First Date is greater than Second Date.");
} else {
alert("Second Date is greater than the First Date.");
}
}
CompareDate();
Other Functions in JavaScript
Changing Date Format
We can use JavaScript code to change or format. You can use the getFullYear(), GetMonth() and getDate() functions to set the date format accordingly.
<script>
var current_date=new Date(); //fetches current date
var set_to=current_date.getFullYear()+"-"+(current_date.getMonth()+1)+"-"+current_date.getDate();
document.write("The format followed is yyyy-dd-mm: "+set_to);
</script>
Changing the datetime format to 'yyyy-dd-mm hh:mm:ss'.
<script>
var current_datetime=new Date(); //fetches current date and time
var set_to=current_datetime.getFullYear()+"-"+(current_datetime.getMonth()+1)+"-
"+current_datetime.getDate()+"
"+current_datetime.getHours()+":"+current_datetime.getMinutes()+":"+current_datetime.getSeconds();
document.write("The format followed is yyyy-dd-mm hh:mm:ss : "+set_to);
</script>
Date Method
There are different Date methods in JavaScript and some of them are listed below;
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- getYear()
- now()
- parse()
- setDate()
Interested in working with a team of expert remote developers? Contact us TODAY!